c语言算法实现二叉树的结构类型定义及算法【包括创建、查找、求高度、遍历、路径、结果显示等等】
时间: 2023-10-20 19:26:24 浏览: 102
下面是基于C语言实现二叉树的结构类型定义及算法:
```
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树结构体
typedef struct node {
int data; // 数据域
struct node* left; // 左子树指针
struct node* right; // 右子树指针
} BTNode;
// 创建二叉树
BTNode* createBinaryTree() {
int data;
scanf("%d", &data);
if (data == -1) { // 输入-1表示当前子树为空
return NULL;
}
BTNode* node = (BTNode*)malloc(sizeof(BTNode));
node->data = data;
node->left = createBinaryTree();
node->right = createBinaryTree();
return node;
}
// 查找二叉树中是否存在指定数据
int searchBinaryTree(BTNode* root, int target) {
if (root == NULL) {
return 0;
}
if (root->data == target) {
return 1;
}
return searchBinaryTree(root->left, target) || searchBinaryTree(root->right, target);
}
// 求二叉树的高度
int getHeight(BTNode* root) {
if (root == NULL) {
return 0;
}
int leftHeight = getHeight(root->left);
int rightHeight = getHeight(root->right);
return leftHeight > rightHeight ? leftHeight + 1 : rightHeight + 1;
}
// 遍历二叉树
void traverseBinaryTree(BTNode* root) {
if (root == NULL) {
return;
}
printf("%d ", root->data);
traverseBinaryTree(root->left);
traverseBinaryTree(root->right);
}
// 路径
void path(BTNode* root, int target, int* arr, int index) {
if (root == NULL) {
return;
}
if (root->data == target) {
arr[index] = root->data;
for (int i = 0; i <= index; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return;
}
arr[index] = root->data;
path(root->left, target, arr, index + 1);
path(root->right, target, arr, index + 1);
}
// 结果显示
void printResult(BTNode* root) {
printf("遍历二叉树结果:");
traverseBinaryTree(root);
printf("\n");
printf("二叉树的高度为:%d\n", getHeight(root));
int target;
printf("请输入查找的数据:");
scanf("%d", &target);
if (searchBinaryTree(root, target)) {
printf("查找成功,数据存在于二叉树中\n");
int arr[100];
printf("从根节点到查找数据的路径为:");
path(root, target, arr, 0);
} else {
printf("查找失败,数据不存在于二叉树中\n");
}
}
int main() {
BTNode* root = createBinaryTree();
printResult(root);
return 0;
}
```
以上代码中,我们定义了二叉树的结构体 `BTNode`,其中包含了数据域 `data` 和左右子树指针 `left` 和 `right`。我们实现了二叉树的创建、查找、求高度、遍历、路径和结果显示等操作。
在 `createBinaryTree` 函数中,我们通过递归的方式创建了二叉树。函数中输入 `-1` 表示当前子树为空。
在 `searchBinaryTree` 函数中,我们通过递归的方式查找二叉树中是否存在指定数据。
在 `getHeight` 函数中,我们通过递归的方式求出了二叉树的高度。
在 `traverseBinaryTree` 函数中,我们通过递归的方式遍历了二叉树,并输出了遍历结果。
在 `path` 函数中,我们通过递归的方式求出了从根节点到指定数据的路径,并输出了结果。
在 `printResult` 函数中,我们将各个操作整合在一起,并输出了最终结果。
最后,在 `main` 函数中,我们创建了二叉树,调用了 `printResult` 函数,并输出了最终结果。
阅读全文
相关推荐
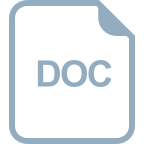
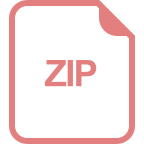
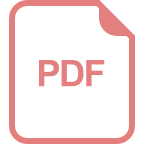
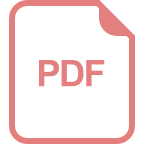
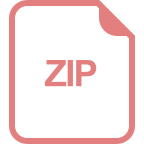
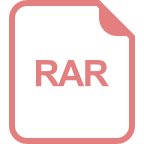
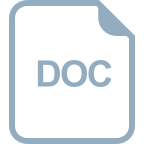
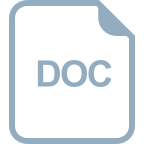
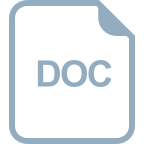
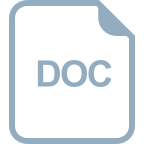
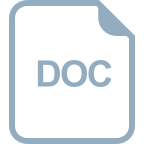
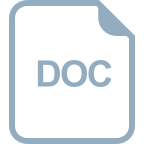
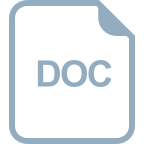
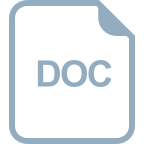