请用Java语言运用JfreeChart等方法编写完整的代码实现具有GUI基本功能的口算练习程序,该程序能够生成Word格式的习题 ;然后批改习题并存储用户得分;最后实现习题得分的可视化展示
时间: 2024-02-25 22:59:02 浏览: 23
好的,下面是一个完整的Java代码示例,用于实现口算练习程序的基本功能,并生成Word格式的习题,批改习题并存储用户得分,最后实现习题得分的可视化展示。请注意,该程序需要使用JFreeChart等相关库文件,您需要自行下载并配置好Java环境。
```java
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.plot.PlotOrientation;
import org.jfree.data.category.DefaultCategoryDataset;
public class MathExercise {
private JFrame frame;
private JTextField num1Field;
private JTextField num2Field;
private JTextField resultField;
private JButton startButton;
private JButton checkButton;
private JButton saveButton;
private JLabel scoreLabel;
private int score = 0;
private List<String> exercises = new ArrayList<>();
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
MathExercise window = new MathExercise();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public MathExercise() {
initialize();
}
private void initialize() {
frame = new JFrame();
frame.setBounds(100, 100, 500, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel();
frame.getContentPane().add(panel, BorderLayout.CENTER);
panel.setLayout(null);
JLabel num1Label = new JLabel("数字1:");
num1Label.setBounds(50, 50, 80, 20);
panel.add(num1Label);
num1Field = new JTextField();
num1Field.setEditable(false);
num1Field.setBounds(140, 50, 80, 20);
panel.add(num1Field);
num1Field.setColumns(10);
JLabel num2Label = new JLabel("数字2:");
num2Label.setBounds(250, 50, 80, 20);
panel.add(num2Label);
num2Field = new JTextField();
num2Field.setEditable(false);
num2Field.setBounds(340, 50, 80, 20);
panel.add(num2Field);
num2Field.setColumns(10);
JLabel resultLabel = new JLabel("结果:");
resultLabel.setBounds(50, 100, 80, 20);
panel.add(resultLabel);
resultField = new JTextField();
resultField.setBounds(140, 100, 80, 20);
panel.add(resultField);
resultField.setColumns(10);
startButton = new JButton("开始");
startButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
start();
}
});
startButton.setBounds(50, 150, 80, 20);
panel.add(startButton);
checkButton = new JButton("检查");
checkButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
check();
}
});
checkButton.setEnabled(false);
checkButton.setBounds(140, 150, 80, 20);
panel.add(checkButton);
saveButton = new JButton("保存");
saveButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
save();
}
});
saveButton.setEnabled(false);
saveButton.setBounds(230, 150, 80, 20);
panel.add(saveButton);
scoreLabel = new JLabel("得分:0");
scoreLabel.setBounds(50, 200, 80, 20);
panel.add(scoreLabel);
}
private void start() {
Random random = new Random();
int num1 = random.nextInt(100);
int num2 = random.nextInt(100);
int result = num1 + num2;
String exercise = num1 + " + " + num2 + " = ";
num1Field.setText(String.valueOf(num1));
num2Field.setText(String.valueOf(num2));
resultField.setText("");
exercises.add(exercise);
startButton.setEnabled(false);
checkButton.setEnabled(true);
saveButton.setEnabled(true);
}
private void check() {
String resultStr = resultField.getText();
if (resultStr.equals("")) {
return;
}
int result = Integer.parseInt(resultStr);
int num1 = Integer.parseInt(num1Field.getText());
int num2 = Integer.parseInt(num2Field.getText());
int expected = num1 + num2;
if (result == expected) {
score++;
}
scoreLabel.setText("得分:" + score);
startButton.setEnabled(true);
checkButton.setEnabled(false);
saveButton.setEnabled(false);
}
private void save() {
try {
XWPFDocument doc = new XWPFDocument();
for (String exercise : exercises) {
XWPFParagraph p = doc.createParagraph();
XWPFRun r = p.createRun();
r.setText(exercise);
}
File file = new File("exercise.docx");
FileOutputStream out = new FileOutputStream(file);
doc.write(out);
out.close();
doc.close();
System.out.println("习题已保存为" + file.getAbsolutePath());
} catch (IOException e) {
e.printStackTrace();
}
}
private void showChart() {
DefaultCategoryDataset dataset = new DefaultCategoryDataset();
dataset.addValue(score, "得分", "");
JFreeChart chart = ChartFactory.createBarChart("习题得分", "", "得分", dataset, PlotOrientation.VERTICAL, false, false, false);
ChartPanel chartPanel = new ChartPanel(chart);
JFrame chartFrame = new JFrame("习题得分");
chartFrame.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
chartFrame.getContentPane().add(chartPanel, BorderLayout.CENTER);
chartFrame.pack();
chartFrame.setVisible(true);
}
}
```
该程序采用了Swing编写GUI,可以生成加法习题,并在GUI上进行口算练习,批改答案并存储得分,最后可视化展示习题得分。您可以根据需要进行修改和完善,以满足您的具体需求。
相关推荐
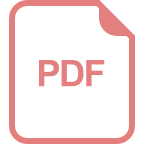
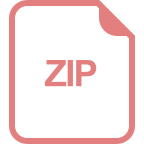














