QCustomPlot实现瀑布图
时间: 2024-01-12 20:17:49 浏览: 134
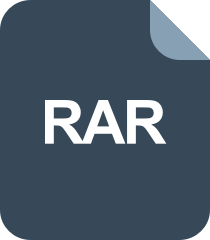
关于QT利用Qcustomplot实现瀑布图的demo

QCustomPlot是一款基于Qt的开源图表库,支持多种图表类型,包括柱状图、折线图、散点图等。要实现瀑布图,可以使用QCustomPlot中的颜色图(QCPColorMap)和直方图(QCPBars)两个元素。
下面是一个简单的瀑布图实现示例:
首先,在Qt Creator中创建一个新的Qt Widgets应用程序项目。在mainwindow.cpp中添加以下代码:
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include "qcustomplot.h"
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
// 创建一个QCustomPlot对象
QCustomPlot *customPlot = new QCustomPlot(this);
// 设置颜色图的数据
int nx = 50, ny = 50;
QVector<double> x(nx), y(ny);
QVector<double> z(nx*ny);
for (int i=0; i<nx; ++i)
x[i] = i/(double)(nx-1)*10;
for (int i=0; i<ny; ++i)
y[i] = i/(double)(ny-1)*10;
for (int i=0; i<nx; ++i)
for (int j=0; j<ny; ++j)
z[i+j*nx] = qSin(x[i]*0.3)*qCos(y[j]*0.3);
// 创建颜色图对象
QCPColorMap *colorMap = new QCPColorMap(customPlot->xAxis, customPlot->yAxis);
colorMap->data()->setSize(nx, ny);
colorMap->data()->setRange(QCPRange(x.first(), x.last()), QCPRange(y.first(), y.last()));
for (int xIndex=0; xIndex<nx; ++xIndex)
for (int yIndex=0; yIndex<ny; ++yIndex)
colorMap->data()->setCell(xIndex, yIndex, z[xIndex+yIndex*nx]);
colorMap->setGradient(QCPColorGradient::gpPolar);
colorMap->rescaleDataRange(true);
customPlot->addPlottable(colorMap);
// 创建直方图对象
QCPBars *bars = new QCPBars(customPlot->xAxis, customPlot->yAxis);
bars->setWidth(1);
bars->setData(x, QVector<double>(nx, 0));
bars->setPen(Qt::NoPen);
bars->setBrush(QColor(20, 20, 20, 100));
customPlot->addPlottable(bars);
// 设置坐标轴标签
customPlot->xAxis->setLabel("X Axis");
customPlot->yAxis->setLabel("Y Axis");
// 设置图例
customPlot->legend->setVisible(true);
customPlot->legend->setFont(QFont("Helvetica", 9));
customPlot->legend->setBrush(QBrush(QColor(255,255,255,230)));
customPlot->legend->setSelectableParts(QCPLegend::spItems); // legend box shall not be selectable, only legend items
// 显示图表
customPlot->replot();
setCentralWidget(customPlot);
}
MainWindow::~MainWindow()
{
delete ui;
}
在上面的代码中,我们首先创建了一个QCustomPlot对象,然后设置颜色图的数据,并创建了一个QCPColorMap对象来显示颜色图。接着,我们创建了一个QCPBars对象来显示直方图。最后,我们设置了坐标轴标签和图例,并显示了图表。
注意,在上面的代码中,我们使用了QVector来存储颜色图的数据。QVector是Qt提供的一种动态数组,可以方便地存储和访问数据。在实际应用中,我们可以根据需要选择不同的数据存储方式。
运行程序,可以看到一个简单的瀑布图。我们可以通过拖动和缩放来查看不同区域的数据。如果需要更多的功能,可以查看QCustomPlot的文档和示例程序。
阅读全文
相关推荐









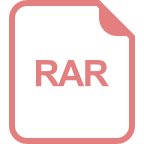






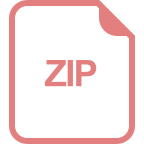