c语言设全班同学有10人,提示输入所有人的成绩后,完成以下操作: (1)统计并输出平均分和最高分、最低分 (2)找出在这10个成绩中超过平均值的那些数字并打印出来。 (3)输入一个学号,查询或修改该同学的成绩
时间: 2024-02-16 17:01:41 浏览: 71
好的,以下是使用C语言实现你的要求的代码示例:
```c
#include <stdio.h>
int main() {
int scores[10];
int sum_score = 0, max_score = 0, min_score = 100;
// 输入所有同学的成绩
for (int i = 0; i < 10; i++) {
printf("请输入第%d个同学的成绩:", i+1);
scanf("%d", &scores[i]);
sum_score += scores[i];
if (scores[i] > max_score) {
max_score = scores[i];
}
if (scores[i] < min_score) {
min_score = scores[i];
}
}
// 统计平均分、最高分、最低分
float avg_score = (float)sum_score / 10;
printf("平均分为:%f,最高分为:%d,最低分为:%d\n", avg_score, max_score, min_score);
// 找出超过平均分的成绩
printf("超过平均分的成绩有:");
for (int i = 0; i < 10; i++) {
if (scores[i] > avg_score) {
printf("%d ", scores[i]);
}
}
printf("\n");
// 查询或修改某个同学的成绩
int student_id;
printf("请输入要查询或修改成绩的同学学号(1-10):");
scanf("%d", &student_id);
if (student_id >= 1 && student_id <= 10) {
int student_index = student_id - 1;
printf("该同学的成绩为:%d\n", scores[student_index]);
printf("是否要修改该同学的成绩?(y/n)");
char choice;
scanf(" %c", &choice);
if (choice == 'y') {
int new_score;
printf("请输入该同学的新成绩:");
scanf("%d", &new_score);
scores[student_index] = new_score;
printf("修改成绩成功!该同学的新成绩为:%d\n", new_score);
}
} else {
printf("输入的学号不合法!\n");
}
return 0;
}
```
这段代码使用了数组存储同学的成绩,通过循环实现了输入、统计、查找和修改操作。与Python示例不同的是,C语言需要手动处理输入和输出,同时需要注意数据类型的转换。
阅读全文
相关推荐
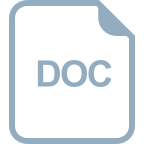
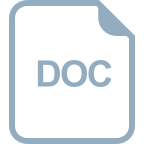
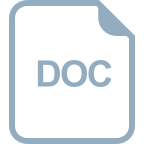
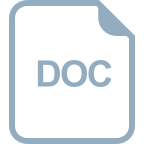
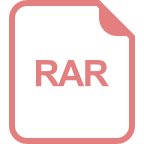
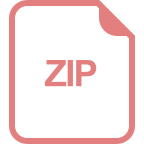
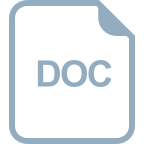