Rewrite the MyStack class in Listing 11.10 to perform a deep copy of the list field.
时间: 2024-05-07 21:16:57 浏览: 12
Sure, here's the modified version of the MyStack class that performs a deep copy of the list field:
```
import java.util.ArrayList;
public class MyStack implements Cloneable {
private ArrayList<Object> list = new ArrayList<>();
public boolean isEmpty() {
return list.isEmpty();
}
public int getSize() {
return list.size();
}
public Object peek() {
return list.get(getSize() - 1);
}
public Object pop() {
Object o = list.get(getSize() - 1);
list.remove(getSize() - 1);
return o;
}
public void push(Object o) {
list.add(o);
}
@Override
public Object clone() {
MyStack cloned = new MyStack();
for (Object o : list) {
cloned.push(o);
}
return cloned;
}
}
```
In the clone() method, a new instance of MyStack is created and populated with a deep copy of the original list field. This ensures that any modifications made to the cloned object don't affect the original object.
相关推荐
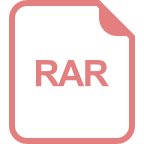
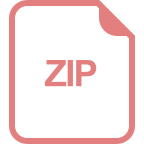














