vue3实现一个竖着滑动年月日的日期选择器组件
时间: 2024-02-21 12:56:52 浏览: 47
好的,这是一个比较复杂的组件,需要考虑多种情况,下面我尝试给出一个简单的实现思路。
首先,我们需要一个容器来放置整个日期选择器,可以用 `div` 元素实现。然后,我们需要一个表头来显示年月,可以用 `div` 元素实现。接着,我们需要一个表格来显示日期,可以用 `table` 元素实现。
在表头中,我们需要显示当前选择的年月,并提供向前和向后翻页的按钮,可以用 `button` 元素实现。在表格中,我们需要显示一整个月的日期,可以用 `td` 元素实现。为了方便处理,我们可以把表格拆分成多个行,每行显示一周的日期。
接下来,我们需要实现滑动功能。可以使用 Vue3 提供的 `@touchstart`、`@touchmove`、`@touchend` 事件,在事件处理函数中计算手指的滑动距离,然后根据距离计算应该滚动到哪个位置。具体实现可以参考如下代码:
```html
<template>
<div class="date-picker" @touchstart="onTouchStart" @touchmove="onTouchMove" @touchend="onTouchEnd">
<div class="header">
<button class="prev" @click="goPrev"><</button>
<div class="title">{{ title }}</div>
<button class="next" @click="goNext">></button>
</div>
<table class="dates">
<thead>
<tr>
<th v-for="(day, index) in days" :key="index">{{ day }}</th>
</tr>
</thead>
<tbody>
<tr v-for="(week, index) in weeks" :key="index">
<td v-for="(date, index) in week" :key="index" :class="{ active: isActive(date) }" @click="select(date)">{{ date }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const days = ['日', '一', '二', '三', '四', '五', '六'];
const weeks = ref([]);
const title = ref('');
const currentDate = ref(new Date());
const startDate = ref(new Date(currentDate.value.getFullYear(), currentDate.value.getMonth(), 1));
const endDate = ref(new Date(currentDate.value.getFullYear(), currentDate.value.getMonth() + 1, 0));
const startY = ref(0);
const endY = ref(0);
const init = () => {
title.value = `${currentDate.value.getFullYear()}年${currentDate.value.getMonth() + 1}月`;
const firstDay = startDate.value.getDay();
const lastDate = endDate.value.getDate();
const prevMonthEndDate = new Date(currentDate.value.getFullYear(), currentDate.value.getMonth(), 0).getDate();
const prevMonthDates = prevMonthEndDate - firstDay + 1;
const nextMonthDates = 42 - lastDate - firstDay;
const dates = [];
for (let i = prevMonthDates; i <= prevMonthEndDate; i++) {
dates.push(i);
}
for (let i = 1; i <= lastDate; i++) {
dates.push(i);
}
for (let i = 1; i <= nextMonthDates; i++) {
dates.push(i);
}
for (let i = 0; i < 6; i++) {
weeks.value[i] = dates.slice(i * 7, (i + 1) * 7);
}
};
const goPrev = () => {
currentDate.value.setMonth(currentDate.value.getMonth() - 1);
startDate.value.setMonth(startDate.value.getMonth() - 1);
endDate.value.setMonth(endDate.value.getMonth() - 1);
init();
};
const goNext = () => {
currentDate.value.setMonth(currentDate.value.getMonth() + 1);
startDate.value.setMonth(startDate.value.getMonth() + 1);
endDate.value.setMonth(endDate.value.getMonth() + 1);
init();
};
const isActive = (date) => {
return date === currentDate.value.getDate();
};
const select = (date) => {
currentDate.value.setDate(date);
init();
};
const onTouchStart = (e) => {
startY.value = e.touches[0].pageY;
};
const onTouchMove = (e) => {
endY.value = e.touches[0].pageY;
const delta = endY.value - startY.value;
if (Math.abs(delta) >= 50) {
if (delta > 0) {
goPrev();
} else {
goNext();
}
startY.value = endY.value;
}
};
const onTouchEnd = () => {
startY.value = 0;
endY.value = 0;
};
init();
return {
days,
weeks,
title,
goPrev,
goNext,
isActive,
select,
onTouchStart,
onTouchMove,
onTouchEnd,
};
},
};
</script>
<style scoped>
.date-picker {
width: 100%;
height: 200px;
overflow: hidden;
}
.header {
display: flex;
justify-content: space-between;
align-items: center;
padding: 10px;
font-size: 16px;
font-weight: bold;
background-color: #f5f5f5;
}
.title {
flex-grow: 1;
text-align: center;
}
.dates {
width: 100%;
border-collapse: collapse;
}
th,
td {
padding: 10px;
text-align: center;
}
.active {
background-color: #409eff;
color: #fff;
}
.prev,
.next {
border: none;
background-color: transparent;
font-size: 16px;
cursor: pointer;
}
.prev:hover,
.next:hover {
color: #409eff;
}
</style>
```
这段代码实现了一个简单的竖着滑动年月日的日期选择器组件,使用了 Vue3 的 Composition API,使用了 `ref` 和 `setup` 函数。具体实现过程中,我们使用了一些计算属性和事件处理函数,实现了日期的显示和滑动功能。
相关推荐
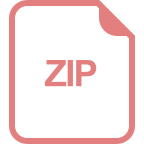
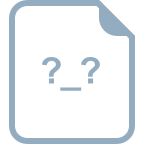














