string str = "To be or not to be"; string[] str2 = str.ToLower().Split(' '); List<string> newStr = new List<string>(); foreach (string item in str2) { if (newStr.Contains(item)) { continue; } else { newStr.Add(item); } } foreach (string item in newStr) { Console.Write(item + ' '); } 改进代码
时间: 2024-02-14 22:07:30 浏览: 70
这段代码的问题在于,它没有考虑到单词之间可能会有标点符号或其他非字母字符,这些字符会导致程序将它们和相邻的单词看成不同的单词。另外,在创建新列表时,可以使用 Linq 中的 Distinct() 方法来去除重复项,而不是手动遍历列表。
以下是改进后的代码:
```
string str = "To be or not to be!";
string[] str2 = str.ToLower().Split(new char[] {' ', ',', '.', '!', '?', ';', ':'}, StringSplitOptions.RemoveEmptyEntries);
List<string> newStr = str2.Distinct().ToList();
foreach (string item in newStr)
{
Console.Write(item + ' ');
}
```
改进后的代码使用 Split() 方法的重载形式,将空格、逗号、句号、感叹号、问号、分号和冒号都作为分隔符,将字符串分割成单词数组。然后使用 Linq 的 Distinct() 方法来去除重复项,并将结果转换为列表。最后遍历列表,输出每个单词。这样可以确保所有单词都能被正确处理。
相关问题
string str = "To be or not to be"; string[] str2 = str.ToLower().Split(' '); List<string> newStr = new List<string>(); foreach (string item in str2) { if (newStr.Contains(item)) { continue; } else { newStr.Add(item); } } foreach (string item in newStr) { Console.Write(item + ' '); }
这段代码的作用是将字符串 "To be or not to be" 中的单词去重,并输出去重后的结果。
这段代码可以进一步优化,使用 LINQ 来简化:
```csharp
string str = "To be or not to be";
var newStr = str.ToLower().Split(' ').Distinct();
Console.WriteLine(string.Join(' ', newStr));
```
这里使用了 ToLower 方法将字符串转换为小写,使用 Split 方法将字符串分割成单词,使用 Distinct 方法去重,最后使用 Join 方法将去重后的单词拼接成字符串并输出。
public static decimal GetSingleTON(string specification) { //sample:2.2KG*5/箱 decimal dm = 1; if (!string.IsNullOrEmpty(specification)) { //step 1 将specification转小写后,按[*]分割 specification = specification.ToLower(); string[] strs = specification.Split('*'); List<decimal> parms = new List<decimal>(); foreach (string str in strs) { if (str.Contains(".")) { string result = Regex.Replace(str, @"[^\d.\d]", ""); //小数 decimal val = decimal.Parse(result); dm = dm * val; } else { string result = System.Text.RegularExpressions.Regex.Replace(str, @"[^0-9]+", ""); //整数 decimal val = decimal.Parse(result); dm = dm * val; } } if (specification.Contains("kg") || specification.Contains("千克") || specification.Contains("公斤")) { dm = dm / 1000; } else if (specification.Contains("g") || specification.Contains("克")) { dm = dm / 1000000; } } return dm; }转换成java代码
公共静态十进制数 GetSingleTON(String specification){
十进制数 dm = new 十进制数(1);
如果(!字符串.isNullOrEmpty(specification)){
specification = specification.toLowerCase();
字符串[] strs = specification.split("*");
List< 十进制数 > parms = new ArrayList< 十进制数 >();
for(字符串 str : strs){
如果(str.contains(".")){
字符串 result = str.replaceAll("[^\\d.\\d]", "");
十进制数 val = new 十进制数(result);
dm = dm.multiply(val);
}else{
字符串 result = str.replaceAll("[^0-9]", "");
十进制数 val = new 十进制数(result);
dm = dm.multiply(val);
}
}
如果(specification.contains("kg") || specification.contains("千克") || specification.contains("公斤")){
dm = dm.divide(new 十进制数(1000));
}else 如果(specification.contains("g") || specification.contains("克")){
dm = dm.divide(new 十进制数(1000000));
}
}
返回 dm;
}
阅读全文
相关推荐
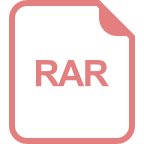
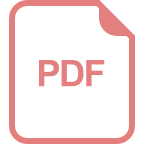
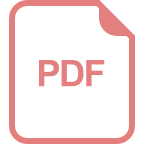
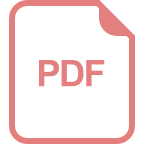
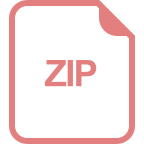
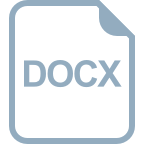
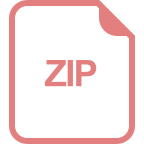
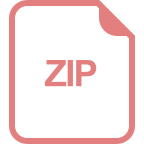
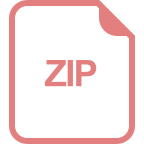
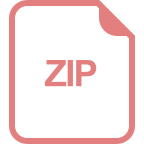
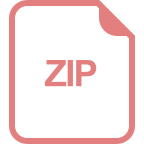
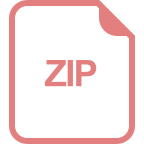
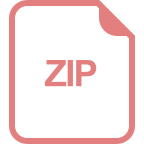