python怎样将dataframe中的两行交换顺序
时间: 2023-02-07 18:41:03 浏览: 133
在Python中可以使用以下方法来交换两行的顺序:
1. 先将要交换的两行提取出来,单独存成两个新的变量。
2. 删除原来的两行。
3. 将两个新变量插入到想要交换到的位置即可。
下面是一个示例代码:
```
import pandas as pd
# 创建一个示例数据框
df = pd.DataFrame({'A': [1, 2], 'B': [3, 4]})
print(df)
# 提取第一行和第二行
row1 = df.iloc[0]
row2 = df.iloc[1]
# 删除原来的两行
df = df.drop([0, 1])
# 将两行插入到想要交换到的位置
df.loc[0] = row2
df.loc[1] = row1
print(df)
```
运行结果如下:
```
A B
0 1 3
1 2 4
A B
0 2 4
1 1 3
```
相关问题
python将两个dataframe的行接在一期
可以使用`pandas`库中的`concat`函数将两个`dataframe`的行接在一起。具体操作步骤如下:
假设有两个`dataframe`,分别为`df1`和`df2`,它们的列名和数据类型都相同,可以按照以下方式将它们的行合并:
```python
import pandas as pd
# 创建两个dataframe
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [7, 8, 9], 'B': [10, 11, 12]})
# 使用concat函数将两个dataframe的行合并
df = pd.concat([df1, df2], ignore_index=True)
# 打印合并后的dataframe
print(df)
```
其中,`ignore_index=True`参数表示在合并后重新为行编号,如果不设置该参数,则会保留原有的行编号。
python怎样将dataframe的一列设为行索引
### 回答1:
可以使用 `set_index()` 函数将 DataFrame 中的某一列设为行索引。
使用方法如下:
```
df.set_index('列名')
```
也可以通过将 `inplace` 参数设为 `True` 来在原有 DataFrame 上进行修改:
```
df.set_index('列名', inplace=True)
```
如果想要将多列设为行索引,可以将这些列名放在一个列表中传给 `set_index()` 函数:
```
df.set_index(['列名1', '列名2'])
```
### 回答2:
在Python中,你可以使用pandas库中的DataFrame的set_index()方法将DataFrame的一列设为行索引。set_index()方法接受一个参数column,该参数指定要设为行索引的列名。
下面是一个示例代码,展示了如何使用set_index()方法将DataFrame的一列设为行索引:
```python
import pandas as pd
# 创建一个示例DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [25, 30, 35, 40],
'City': ['New York', 'London', 'Paris', 'Tokyo']}
df = pd.DataFrame(data)
# 将'Name'列设为行索引
df.set_index('Name', inplace=True)
print(df)
```
运行上述代码后,输出将是:
```
Age City
Name
Alice 25 New York
Bob 30 London
Charlie 35 Paris
David 40 Tokyo
```
在这个例子中,我们使用set_index()方法将DataFrame的'Name'列设为行索引。参数inplace=True表示直接在原始DataFrame上进行修改,而不是返回一个新的DataFrame对象。
希望这个回答对你有所帮助!
### 回答3:
要将dataframe的一列设为行索引,可以使用pandas库中的set_index()函数。
set_index()函数允许我们指定一个或多个列名来替换当前索引,将这些列作为新的索引。在这种情况下,我们只需要指定要设置为索引的列名即可。
例如,假设我们有以下的dataframe:
```
姓名 年龄 性别
0 甲 25 男
1 乙 30 女
2 丙 28 男
```
现在我们想将"姓名"列作为索引,则可以使用set_index()函数:
```python
import pandas as pd
df = pd.DataFrame({'姓名': ['甲', '乙', '丙'],
'年龄': [25, 30, 28],
'性别': ['男', '女', '男']})
df.set_index('姓名', inplace=True)
```
设置inplace=True参数可以使修改直接在原始dataframe上进行。
执行以上代码后,dataframe将变为:
```
年龄 性别
姓名
甲 25 男
乙 30 女
丙 28 男
```
现在"姓名"列已经成为了行索引。
相关推荐
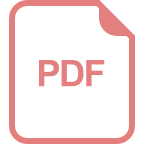
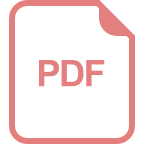












