fortran和C++混编示例
时间: 2023-07-11 14:47:21 浏览: 57
Fortran和C++可以通过一些接口来实现混编,以下是一个简单的示例:
首先是Fortran程序,其中包含了一个Fortran函数和一个接口:
```
! sample.f90
module sample
implicit none
contains
! Fortran函数
real function func(x)
real, intent(in) :: x
func = x**2 + 2*x + 1
end function func
! 接口
interface
real function cplusplus_func(x) bind(C, name="cplusplus_func")
import :: C_DOUBLE
real(C_DOUBLE), intent(in) :: x
end function cplusplus_func
end interface
end module sample
```
接着是C++程序,其中包含了一个C++函数和一个接口:
```
// sample.cpp
#include <iostream>
using namespace std;
extern "C" {
// C++函数
double cplusplus_func(double x) {
return x*x + 2*x + 1;
}
// 接口
extern double func_(double *x);
}
int main() {
double x = 2.0;
// 调用C++函数
double y = cplusplus_func(x);
cout << "C++ function: " << y << endl;
// 调用Fortran函数
double z = func_(&x);
cout << "Fortran function: " << z << endl;
return 0;
}
```
在C++程序中,我们使用了`extern "C"`来声明C++函数和接口,以便在链接时能够正确地找到它们。在主函数中,我们首先调用了一个C++函数,然后通过接口调用了一个Fortran函数。
编译Fortran程序时,需要使用`-c`选项生成静态库文件:
```
$ gfortran -c sample.f90
```
编译C++程序时,需要链接静态库文件,并使用`-lstdc++`选项链接C++标准库:
```
$ g++ sample.cpp sample.o -lstdc++
```
运行程序,可以得到如下输出:
```
C++ function: 9
Fortran function: 9
```
说明我们成功地实现了Fortran和C++的混编。
相关推荐
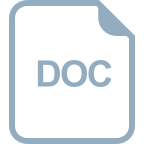














