java 如果一个自定义异常,除了code和msg,还有channel或者其它的属性,怎么让它抛出时,让前端获取到
时间: 2024-11-09 19:26:22 浏览: 6
在Java中,如果你想要创建一个自定义异常并包含额外属性如`channel`等,你可以这样做:
首先,创建一个新的类,继承自`Exception`或它的子类,比如`RuntimeException`或`IOException`(取决于你的业务场景)。例如:
```java
public class CustomException extends RuntimeException {
private int code;
private String msg;
private String channel;
// 构造函数接收所有属性
public CustomException(int code, String msg, String channel) {
super(msg); // 父类构造函数,传入错误消息
this.code = code;
this.msg = msg;
this.channel = channel;
}
// 获取属性的方法
public int getCode() {
return code;
}
public String getMsg() {
return msg;
}
public String getChannel() {
return channel;
}
@Override
public String toString() {
return "CustomException{" +
"code=" + code +
", msg='" + msg + '\'' +
", channel='" + channel + '\'' +
'}';
}
}
```
当你需要抛出这个异常时,可以直接实例化并传入所需的参数:
```java
try {
// 一些可能导致异常的操作
} catch (Exception e) {
if (e instanceof CustomException) {
CustomException customException = (CustomException) e;
// 获取并处理异常信息
int errorCode = customException.getCode();
String errorMsg = customException.getMsg();
String channel = customException.getChannel();
// 可能的前端交互代码
throw new WebException(errorCode, errorMsg, channel);
} else {
// 其他非自定义异常处理
}
}
```
当这个自定义异常被抛出并通过网络传递给前端时,前端通常会接收到一个包含了`code`、`msg`和`channel`的响应对象,并可以根据这些信息进行相应的错误处理。注意这里假设前端可能有一个名为`WebException`的异常类用于封装从服务器返回的信息。
阅读全文
相关推荐
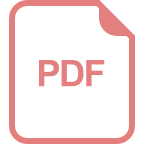
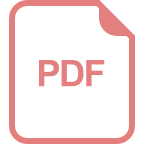
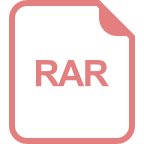
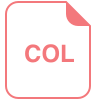
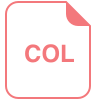
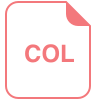
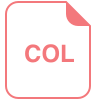
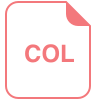
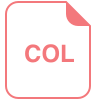
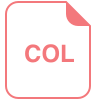
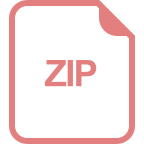
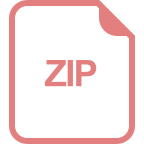
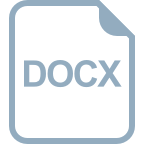