Java中Lable的布局
时间: 2023-12-06 08:40:15 浏览: 74
在Java中,Label可以使用布局管理器来设置其位置和大小。以下是几种常见的布局:
1. BorderLayout:使用BorderLayout布局管理器时,Label可以放置在North、South、East、West和Center中的任何一个位置。
2. FlowLayout:使用FlowLayout布局管理器时,Label按照从左到右、从上到下的顺序排列。
3. GridLayout:使用GridLayout布局管理器时,Label可以放置在一个网格中的任何一个位置。
4. GridBagLayout:使用GridBagLayout布局管理器时,可以更加灵活地设置Label的位置和大小。
5. BoxLayout:使用BoxLayout布局管理器时,可以按照水平或垂直方向排列Label。
需要注意的是,不同的布局管理器适用于不同的场景,需要根据实际需求进行选择。
相关问题
Java写一个窗口,要求使用流式布局, 三个box,一个box1放lable,box2放输入内容,box1,box2放在baseBox里,确认和取消按钮放在basebox下面,只实现布局,不实现逻辑
好的,以下是Java代码实现窗口流式布局的要求:
```java
import javax.swing.*;
import java.awt.*;
public class MyWindow extends JFrame {
private JLabel label;
private JTextField textField;
private JButton confirmBtn, cancelBtn;
public MyWindow() {
init();
setTitle("My Window");
setSize(400, 200);
setLocationRelativeTo(null);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private void init() {
label = new JLabel("输入内容:");
textField = new JTextField(20);
confirmBtn = new JButton("确认");
cancelBtn = new JButton("取消");
// 创建三个Box,一个放Label,一个放输入内容,一个放确认和取消按钮
Box box1 = Box.createHorizontalBox();
Box box2 = Box.createHorizontalBox();
Box box3 = Box.createHorizontalBox();
// 往第一个Box里添加Label
box1.add(label);
// 往第二个Box里添加输入框
box2.add(textField);
// 往第三个Box里添加确认和取消按钮
box3.add(confirmBtn);
box3.add(Box.createHorizontalStrut(20)); // 添加20像素的水平间距
box3.add(cancelBtn);
// 创建一个基础Box,把前面的三个Box添加进去
Box baseBox = Box.createVerticalBox();
baseBox.add(Box.createVerticalStrut(20)); // 添加20像素的垂直间距
baseBox.add(box1);
baseBox.add(Box.createVerticalStrut(10)); // 添加10像素的垂直间距
baseBox.add(box2);
baseBox.add(Box.createVerticalStrut(20)); // 添加20像素的垂直间距
baseBox.add(box3);
// 设置窗口布局为流式布局
setLayout(new FlowLayout());
// 把基础Box添加到窗口中
add(baseBox);
}
public static void main(String[] args) {
new MyWindow();
}
}
```
注:以上代码只实现了窗口的布局,未实现确认和取消按钮的逻辑。
Java写一个窗口,要求使用流式布局,学号,姓名,性别,班级下拉列表,邮箱,地址,电话, 三个box,一个box1放lable,box2放输入内容,box1,box2放在baseBox里,确认和取消按钮放在basebox下面,只实现布局,不实现逻辑
好的,以下是Java代码实现窗口流式布局的要求:
```java
import javax.swing.*;
import java.awt.*;
public class MyWindow extends JFrame {
private JLabel idLabel, nameLabel, genderLabel, classLabel, emailLabel, addressLabel, phoneLabel;
private JTextField idField, nameField, emailField, addressField, phoneField;
private JComboBox<String> genderComboBox, classComboBox;
private JButton confirmBtn, cancelBtn;
public MyWindow() {
init();
setTitle("My Window");
setSize(400, 400);
setLocationRelativeTo(null);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private void init() {
idLabel = new JLabel("学号:");
nameLabel = new JLabel("姓名:");
genderLabel = new JLabel("性别:");
classLabel = new JLabel("班级:");
emailLabel = new JLabel("邮箱:");
addressLabel = new JLabel("地址:");
phoneLabel = new JLabel("电话:");
idField = new JTextField(20);
nameField = new JTextField(20);
emailField = new JTextField(20);
addressField = new JTextField(20);
phoneField = new JTextField(20);
genderComboBox = new JComboBox<>(new String[]{"男", "女"});
classComboBox = new JComboBox<>(new String[]{"软件工程1班", "软件工程2班", "软件工程3班"});
confirmBtn = new JButton("确认");
cancelBtn = new JButton("取消");
// 创建三个Box,一个放Label,一个放输入内容,一个放确认和取消按钮
Box box1 = Box.createVerticalBox();
Box box2 = Box.createVerticalBox();
Box box3 = Box.createHorizontalBox();
// 往第一个Box里添加各个Label
box1.add(idLabel);
box1.add(Box.createVerticalStrut(10)); // 添加10像素的垂直间距
box1.add(nameLabel);
box1.add(Box.createVerticalStrut(10));
box1.add(genderLabel);
box1.add(Box.createVerticalStrut(10));
box1.add(classLabel);
box1.add(Box.createVerticalStrut(10));
box1.add(emailLabel);
box1.add(Box.createVerticalStrut(10));
box1.add(addressLabel);
box1.add(Box.createVerticalStrut(10));
box1.add(phoneLabel);
// 往第二个Box里添加各个输入框和下拉列表
box2.add(idField);
box2.add(Box.createVerticalStrut(10));
box2.add(nameField);
box2.add(Box.createVerticalStrut(10));
box2.add(genderComboBox);
box2.add(Box.createVerticalStrut(10));
box2.add(classComboBox);
box2.add(Box.createVerticalStrut(10));
box2.add(emailField);
box2.add(Box.createVerticalStrut(10));
box2.add(addressField);
box2.add(Box.createVerticalStrut(10));
box2.add(phoneField);
// 往第三个Box里添加确认和取消按钮
box3.add(confirmBtn);
box3.add(Box.createHorizontalStrut(20)); // 添加20像素的水平间距
box3.add(cancelBtn);
// 创建一个基础Box,把前面的三个Box添加进去
Box baseBox = Box.createVerticalBox();
baseBox.add(Box.createVerticalStrut(20)); // 添加20像素的垂直间距
baseBox.add(box1);
baseBox.add(Box.createVerticalStrut(10)); // 添加10像素的垂直间距
baseBox.add(box2);
baseBox.add(Box.createVerticalStrut(20)); // 添加20像素的垂直间距
baseBox.add(box3);
// 设置窗口布局为流式布局
setLayout(new FlowLayout());
// 把基础Box添加到窗口中
add(baseBox);
}
public static void main(String[] args) {
new MyWindow();
}
}
```
注:以上代码只实现了窗口的布局,未实现确认和取消按钮的逻辑。
阅读全文
相关推荐
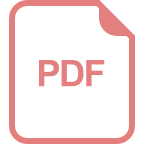
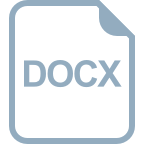
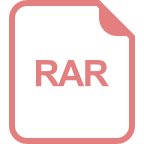
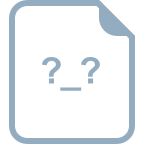
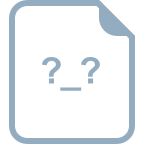
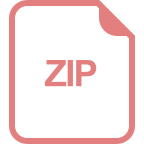



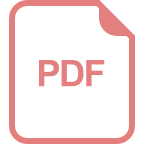
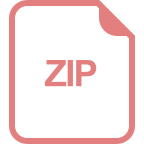
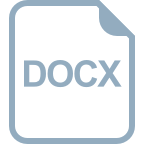
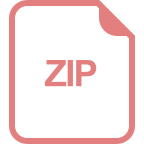