如何用javafx实现窗口内的一个命令行终端。请给出代码实例
时间: 2024-06-10 16:10:20 浏览: 13
以下是一个基本的JavaFX命令行终端的示例代码:
```
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextArea;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class CommandLineTerminal extends Application {
private TextArea outputArea;
@Override
public void start(Stage primaryStage) throws Exception {
outputArea = new TextArea();
outputArea.setEditable(false);
TextField inputField = new TextField();
VBox root = new VBox(outputArea, inputField);
Scene scene = new Scene(root, 400, 300);
primaryStage.setScene(scene);
primaryStage.show();
inputField.setOnAction(event -> {
String command = inputField.getText();
inputField.clear();
outputArea.appendText("$ " + command + "\n");
executeCommand(command);
});
}
private void executeCommand(String command) {
try {
Process process = Runtime.getRuntime().exec(command);
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
outputArea.appendText(line + "\n");
}
} catch (IOException e) {
outputArea.appendText("Error executing command: " + e.getMessage() + "\n");
}
}
public static void main(String[] args) {
launch(args);
}
}
```
该应用程序使用一个`VBox`布局来将一个`TextArea`和一个`TextField`垂直排列在一起。`TextArea`用于输出命令行的结果,而`TextField`用于接受用户的命令输入。
当用户在`TextField`中按下回车键时,应用程序将执行输入的命令,并将结果输出到`TextArea`中。在执行命令时,应用程序使用Java的`Runtime.getRuntime().exec()`方法来启动一个新的进程,并通过`BufferedReader`读取该进程的标准输出流。
此示例只是一个简单的终端模拟器,不具有完整的功能,例如支持多个命令和命令历史记录等。但它可以作为一个基础,用于构建更高级的终端应用程序。
相关推荐
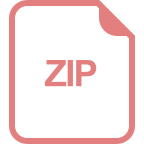














