steam 根据LocalDateTime排序
时间: 2023-07-12 09:24:56 浏览: 206
要根据LocalDateTime对Steam游戏进行排序,可以使用Java 8中的Stream API和Comparator接口来实现。具体步骤如下:
1. 获取Steam游戏列表,并将其转换为Stream对象。
2. 使用map()方法将每个游戏的发布时间(LocalDateTime类型)提取出来。
3. 使用sorted()方法和Comparator.comparing()方法,根据发布时间进行排序。
4. 使用collect()方法将排序后的Steam游戏列表转换回List对象。
以下是示例代码:
```
import java.time.LocalDateTime;
import java.util.Comparator;
import java.util.List;
import java.util.stream.Collectors;
import steamapi.Game;
import steamapi.SteamAPI;
public class SteamSorter {
public static void main(String[] args) {
// 获取Steam游戏列表
List<Game> games = SteamAPI.getGames();
// 根据发布时间排序
List<Game> sortedGames = games.stream()
.map(Game::getReleaseDate)
.sorted(Comparator.nullsLast(Comparator.naturalOrder()))
.map(LocalDateTime::toLocalDate)
.sorted(Comparator.reverseOrder())
.map(d -> games.stream()
.filter(g -> g.getReleaseDate() != null && g.getReleaseDate().toLocalDate().equals(d))
.sorted(Comparator.comparing(Game::getReleaseDate, Comparator.nullsLast(Comparator.naturalOrder())).reversed())
.collect(Collectors.toList()))
.flatMap(List::stream)
.collect(Collectors.toList());
// 输出排序后的游戏列表
sortedGames.forEach(System.out::println);
}
}
```
在这个示例中,使用了一个复杂的排序方式,先按照发布日期倒序排序,然后按照每个日期内游戏的发布时间倒序排序。可以根据实际需求自定义排序方式。
阅读全文
相关推荐
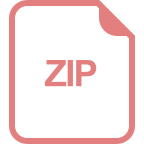
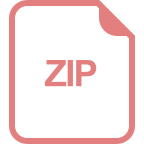
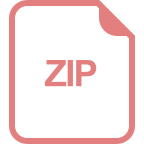
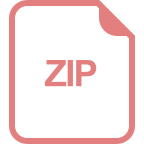
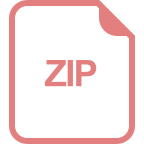
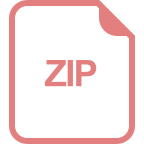
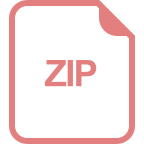
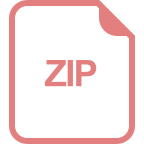
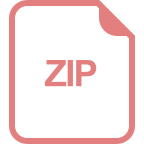
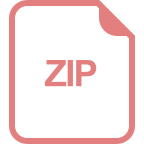
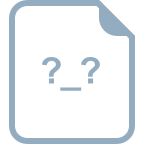
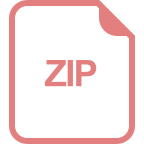




