java根据数据库里的学生成绩查询学生姓名和课程
时间: 2023-11-24 14:26:34 浏览: 125
好的,您可以参考以下代码实现JAVA根据数据库里的学生成绩查询学生姓名和课程的功能:
```java
import java.sql.*;
public class QueryNameAndCourse {
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 1. 加载数据库驱动
Class.forName("com.mysql.jdbc.Driver");
// 2. 创建数据库连接
String url = "jdbc:mysql://localhost:3306/mydb";
String username = "root";
String password = "123456";
conn = DriverManager.getConnection(url, username, password);
// 3. 创建Statement对象
stmt = conn.createStatement();
// 4. 执行SQL语句
String sql = "SELECT student.name, course.name FROM student_score " +
"INNER JOIN student ON student_score.student_id = student.id " +
"INNER JOIN course ON student_score.course_id = course.id";
rs = stmt.executeQuery(sql);
// 5. 处理查询结果
while (rs.next()) {
String studentName = rs.getString(1);
String courseName = rs.getString(2);
System.out.println("学生姓名:" + studentName + ",课程:" + courseName);
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 6. 释放资源
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
其中,需要替换的部分如下:
- 数据库连接信息(url、username、password)
请根据实际情况进行替换。另外,需要注意的是,该查询语句是使用INNER JOIN关键字连接多张表进行查询的,需要确保student_score表中的student_id和course_id字段能够正确地和student表和course表中的id字段匹配。
阅读全文
相关推荐
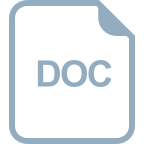
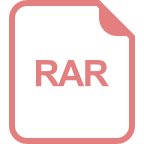
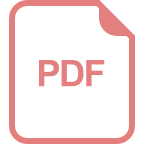
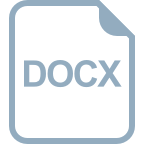
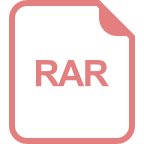
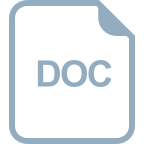
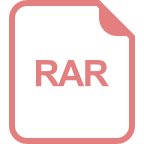
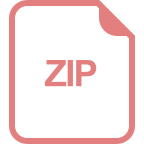
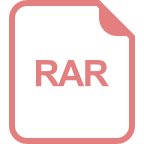
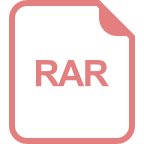
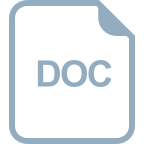
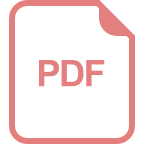
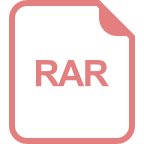
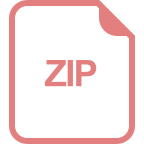
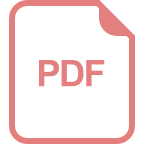
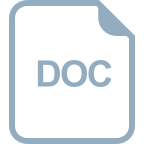
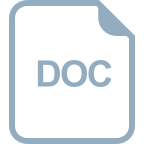