C语言把树的遍历转存入字符串数组
时间: 2023-07-05 07:24:21 浏览: 102
在C语言中,可以通过递归实现树的遍历,并将遍历结果存入字符串数组中。以下是一个示例程序,它将二叉树的中序遍历结果存入字符串数组中:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
// 二叉树结构体
typedef struct TreeNode {
int data;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 创建二叉树
TreeNode* createTree() {
int data;
scanf("%d", &data);
if (data == -1) {
return NULL;
}
TreeNode *root = (TreeNode*)malloc(sizeof(TreeNode));
root->data = data;
root->left = createTree();
root->right = createTree();
return root;
}
// 中序遍历二叉树,并将结果存入字符串数组中
void inorderTraversal(TreeNode *root, char **strings, int *index) {
if (root == NULL) {
return;
}
inorderTraversal(root->left, strings, index);
char str[MAX_SIZE];
sprintf(str, "%d", root->data);
strings[*index] = (char*)malloc(strlen(str) + 1);
strcpy(strings[*index], str);
(*index)++;
inorderTraversal(root->right, strings, index);
}
int main() {
TreeNode *root = createTree();
char *strings[MAX_SIZE];
int index = 0;
inorderTraversal(root, strings, &index);
printf("Inorder Traversal: ");
for (int i=0; i<index; i++) {
printf("%s ", strings[i]);
}
printf("\n");
return 0;
}
```
在该程序中,我们使用 `createTree` 函数创建一个二叉树。然后,我们定义了一个字符串数组 `strings`,用于存储遍历结果。在 `inorderTraversal` 函数中,我们使用递归实现了中序遍历,并将遍历结果存入字符串数组中。最后,我们使用 `printf` 函数输出遍历结果。注意,在程序结束时,需要手动释放字符串数组中每个字符串的内存。
阅读全文
相关推荐
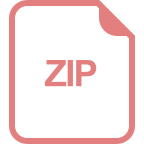
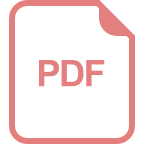
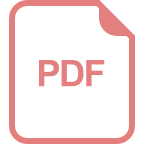
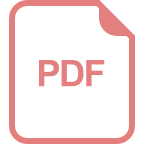
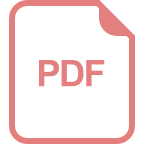
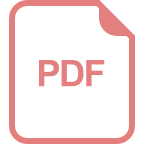
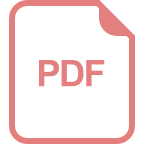
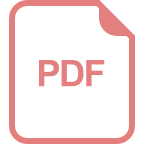