对图片进行seam-carving代码
时间: 2023-05-15 19:04:11 浏览: 117
Seam-carving是一种图像处理技术,它可以对图片进行尺寸调整而不损失图像的重要信息。实现该技术的主要步骤是确定图像中的最小能量路径(seam)并将路径中的像素删除或插入来改变图像的尺寸。以下是一个简单的seam-carving代码实现。
首先,我们需要计算图像的能量矩阵。能量可以用图像中像素的梯度来计算。在本例中,我们采用Sobel算子计算像素梯度。代码如下:
```python
import numpy as np
from scipy.ndimage import convolve
def calc_energy(img):
gray = np.average(img, axis=2)
h_kernel = np.array([[-1, 0, 1], [-2, 0, 2], [-1, 0, 1]])
v_kernel = h_kernel.T
h_grad = np.abs(convolve(gray, h_kernel))
v_grad = np.abs(convolve(gray, v_kernel))
energy = h_grad + v_grad
return energy
```
在得到能量矩阵后,我们需要找出最小能量路径。在本例中,我们使用动态规划算法来找到路径。代码如下:
```python
def find_seam(energy):
M, N = energy.shape
seam = np.zeros(M, dtype=int)
# 初始化路径矩阵
path = np.zeros((M, N), dtype=int)
path[0, :] = energy[0, :]
# 动态规划,计算每个像素的最小能量路径
for i in range(1, M):
for j in range(N):
if j == 0:
idx = np.argmin(path[i - 1, j:j + 2])
seam[i] = j + idx
path[i, j] = energy[i, j] + path[i - 1, j + idx]
elif j == N - 1:
idx = np.argmin(path[i - 1, j - 1:j + 1])
seam[i] = j - 1 + idx
path[i, j] = energy[i, j] + path[i - 1, j - 1 + idx]
else:
idx = np.argmin(path[i - 1, j - 1:j + 2])
seam[i] = j - 1 + idx
path[i, j] = energy[i, j] + path[i - 1, j - 1 + idx]
return seam
```
最后,我们需要利用找到的最小能量路径来缩小或者扩大图像。代码如下:
```python
def seam_carving(img, out_size):
in_h, in_w = img.shape[:2]
out_h, out_w = out_size
# 计算图像能量矩阵
energy = calc_energy(img)
if in_w > out_w:
# 图像宽度缩小
for i in range(in_w - out_w):
# 找到最小能量路径
seam = find_seam(energy)
# 将路径上的像素删除
for j in range(in_h):
img[j, seam[j]:-1] = img[j, seam[j]+1:]
energy[j, seam[j]:-1] = energy[j, seam[j]+1:]
if seam[j] > 0:
energy[j, seam[j]-1] = calc_energy(img[j:j+1, seam[j]-1:seam[j]])[0, 0]
if seam[j] < in_w - 2:
energy[j, seam[j]] = calc_energy(img[j:j+1, seam[j]:seam[j]+1])[0, 0]
img = img[:, :-1]
energy = energy[:, :-1]
elif in_w < out_w:
# 图像宽度扩大
for i in range(out_w - in_w):
# 找到最小能量路径
seam = find_seam(energy)
# 插入新像素
mask = np.ones((in_h, in_w), dtype=bool)
for j in range(in_h):
mask[j, seam[j]] = False
img_new = np.zeros((in_h, in_w + 1, 3))
img_new[:, :-1][mask] = img[mask]
for j in range(in_h):
if seam[j] == 0:
img_new[j, seam[j]] = img[j, seam[j]]
else:
img_new[j, seam[j]] = (img[j, seam[j] - 1] + img[j, seam[j]]) / 2
img_new[j, seam[j] + 1:] = img[j, seam[j]:]
img = img_new.copy()
# 更新能量矩阵
energy = calc_energy(img)
return img
```
通过上述代码,我们可以实现一个简单的seam-carving算法,对图像进行尺寸调整。
阅读全文
相关推荐
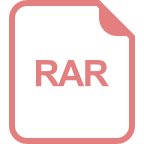
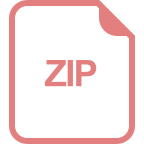
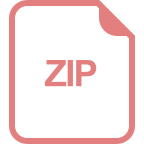
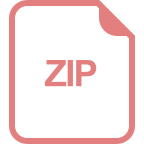
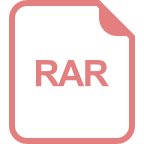
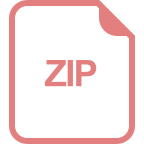
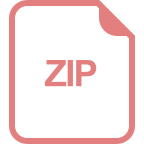
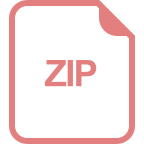
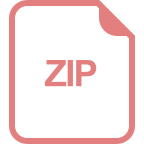
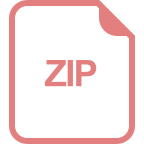
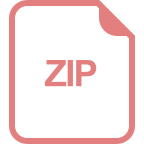
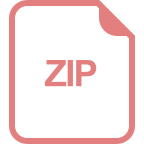
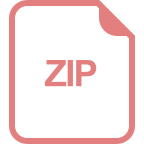
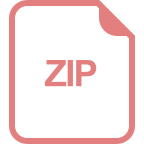
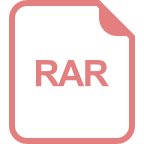
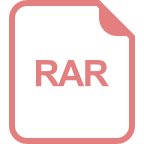
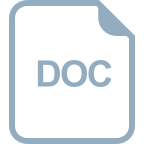