vue 画一个ip地址输入
时间: 2024-02-26 15:57:17 浏览: 110
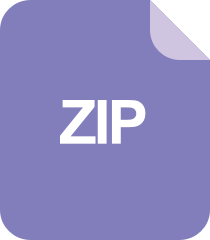
vue-ip-input-由vuejs实现的ip输入。-Vue.js开发
你使用 Vue 组件来实现 IP 地址输入框,以下是一个简单的示例:
```html
<template>
<div>
<input v-model="octet1" type="text" maxlength="3" @input="handleInput" />
.
<input v-model="octet2" type="text" maxlength="3" @input="handleInput" />
.
<input v-model="octet3" type="text" maxlength="3" @input="handleInput" />
.
<input v-model="octet4" type="text" maxlength="3" @input="handleInput" />
</div>
</template>
<script>
export default {
data() {
return {
octet1: '',
octet2: '',
octet3: '',
octet4: '',
};
},
methods: {
handleInput(event) {
const input = event.target;
const maxLength = parseInt(input.getAttribute('maxlength'));
const value = input.value;
// 如果输入的字符数超过了最大长度,则截断输入
if (value.length > maxLength) {
input.value = value.slice(0, maxLength);
}
// 将输入的值限制在 0-255 范围内
const intValue = parseInt(value);
if (isNaN(intValue) || intValue < 0 || intValue > 255) {
input.value = '';
}
// 将输入的值赋值给对应的变量
const name = input.getAttribute('v-model');
this[name] = input.value;
},
},
watch: {
// 监听变量变化,合并为 IP 地址
octet1(newValue, oldValue) {
if (newValue !== '' && (newValue !== oldValue || oldValue === '')) {
this.$emit('input', `${this.octet1}.${this.octet2}.${this.octet3}.${this.octet4}`);
}
},
octet2(newValue, oldValue) {
if (newValue !== '' && (newValue !== oldValue || oldValue === '')) {
this.$emit('input', `${this.octet1}.${this.octet2}.${this.octet3}.${this.octet4}`);
}
},
octet3(newValue, oldValue) {
if (newValue !== '' && (newValue !== oldValue || oldValue === '')) {
this.$emit('input', `${this.octet1}.${this.octet2}.${this.octet3}.${this.octet4}`);
}
},
octet4(newValue, oldValue) {
if (newValue !== '' && (newValue !== oldValue || oldValue === '')) {
this.$emit('input', `${this.octet1}.${this.octet2}.${this.octet3}.${this.octet4}`);
}
},
},
};
</script>
```
上面的代码定义了一个名为 `IPInput` 的组件,使用了 `v-model` 来双向绑定 IP 地址。用户在输入框中输入 IP 地址时,组件会将输入的值限制在 0-255 范围内,并且将输入的值合并为完整的 IP 地址,然后通过 `$emit` 方法向父组件传递 IP 地址值。在父组件中,你可以使用 `v-model` 来绑定 IP 地址的值,就像这样:
```html
<template>
<div>
<IPInput v-model="ipAddress" />
</div>
</template>
<script>
import IPInput from './IPInput.vue';
export default {
data() {
return {
ipAddress: '',
};
},
components: {
IPInput,
},
};
</script>
```
这样,你就可以在父组件中使用 `ipAddress` 变量来获取或设置 IP 地址的值了。
阅读全文
相关推荐
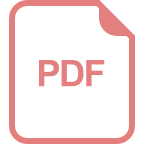
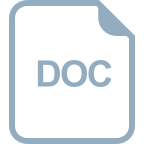
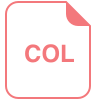
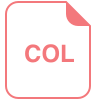
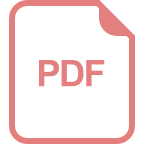
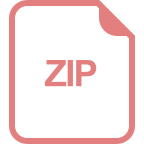
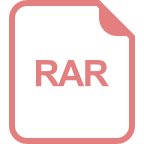
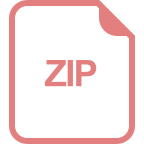
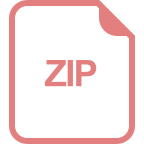
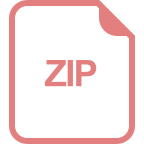
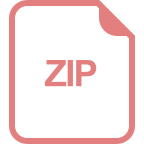
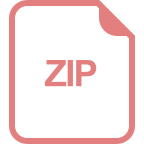
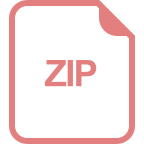
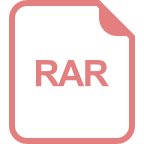
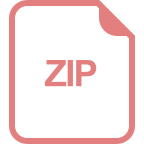
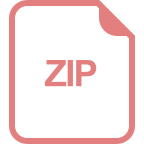