golang编写http请求
时间: 2023-11-02 21:12:29 浏览: 118
示例代码:
```go
package main
import (
"fmt"
"net/http"
"io/ioutil"
)
func main() {
// 发送 GET 请求
response, err := http.Get("https://example.com")
if err != nil {
fmt.Println("请求失败:", err)
return
}
defer response.Body.Close()
// 读取响应内容
body, err := ioutil.ReadAll(response.Body)
if err != nil {
fmt.Println("读取响应失败:", err)
return
}
fmt.Println(string(body))
// 发送 POST 请求
request, err := http.NewRequest("POST", "https://example.com", nil)
if err != nil {
fmt.Println("创建请求失败:", err)
return
}
client := &http.Client{}
response, err = client.Do(request)
if err != nil {
fmt.Println("请求失败:", err)
return
}
defer response.Body.Close()
// 读取响应内容
body, err = ioutil.ReadAll(response.Body)
if err != nil {
fmt.Println("读取响应失败:", err)
return
}
fmt.Println(string(body))
}
```
该示例中,发送 GET 请求使用了 `http.Get(url string)` 函数,发送 POST 请求使用了 `http.NewRequest(method, url string, body io.Reader)` 函数和 `client.Do(request *http.Request)` 方法。读取响应内容使用了 `ioutil.ReadAll(response.Body)` 函数。
阅读全文
相关推荐
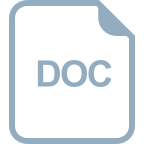
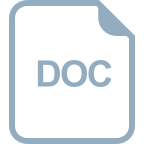
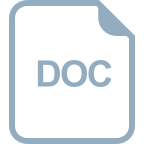
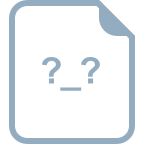
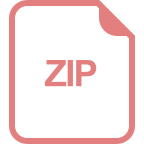
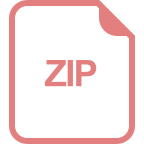
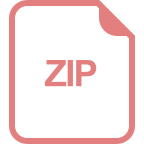
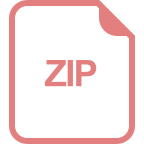
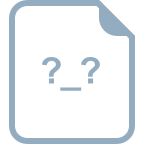
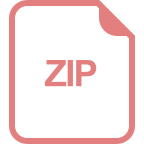
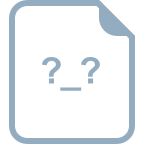
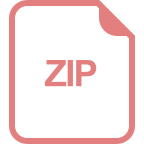
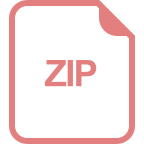
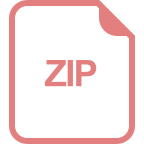
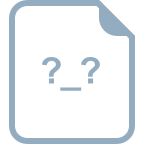
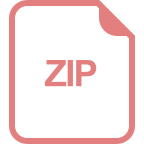
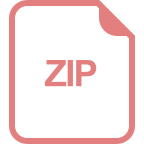
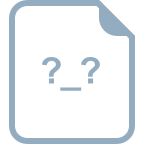
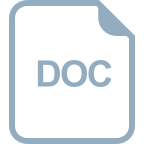