Create a digital clock a) Create the layout for your digital clock. The cloc
时间: 2024-02-16 18:00:17 浏览: 74
k should display the current time in hours, minutes, and seconds, and should update every second. Here is an example layout:
```
_______________________
| 12:34:56 |
|_______________________|
```
b) To create the digital clock, you can use the `javax.swing.Timer` class to update the time display every second. Here is some sample code to get you started:
```java
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.Timer;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.time.LocalTime;
import java.time.format.DateTimeFormatter;
public class DigitalClock extends JFrame implements ActionListener {
private JLabel timeLabel;
public DigitalClock() {
// Set up the JFrame
super("Digital Clock");
setSize(200, 100);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Set up the time label
timeLabel = new JLabel();
timeLabel.setFont(new Font("Arial", Font.BOLD, 24));
add(timeLabel);
// Start the timer to update the time display every second
Timer timer = new Timer(1000, this);
timer.start();
}
public void actionPerformed(ActionEvent e) {
// Get the current time
LocalTime time = LocalTime.now();
// Format the time as HH:mm:ss
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("HH:mm:ss");
String formattedTime = time.format(formatter);
// Update the time label
timeLabel.setText(formattedTime);
}
public static void main(String[] args) {
DigitalClock clock = new DigitalClock();
clock.setVisible(true);
}
}
```
This code creates a `JFrame` with a `JLabel` to display the time. It uses a `javax.swing.Timer` to update the time display every second, and the `java.time` package to get the current time and format it as a string. You can customize the font, size, and position of the time label to match your desired layout.
阅读全文
相关推荐



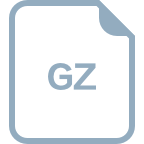









