SimBert,请用标准示例实现以上模型,尽量使用pytorch,并逐行代码注释,并逐层递进通俗易懂且简练的说明模型中使用的原理技术,让一个NLP新手对以上模型的掌握程度达到NLP开发工程师的水平!
时间: 2024-04-17 21:24:58 浏览: 34
抱歉,目前尚无SimBERT的标准示例。SimBERT是一种基于BERT模型的相似度匹配模型,它通过对两个句子进行编码并计算它们之间的相似度来解决句子相似度任务。以下是一个通用的示例,使用BERT模型进行句子相似度匹配:
```python
import torch
import torch.nn as nn
from transformers import BertModel, BertTokenizer
# 加载预训练的BERT模型和分词器
model_name = 'bert-base-uncased'
tokenizer = BertTokenizer.from_pretrained(model_name)
bert_model = BertModel.from_pretrained(model_name)
# 定义句子相似度匹配模型类
class SentenceSimilarity(nn.Module):
def __init__(self, bert_model):
super(SentenceSimilarity, self).__init__()
self.bert = bert_model
self.fc = nn.Linear(bert_model.config.hidden_size, 1)
def forward(self, input_ids_1, attention_mask_1, input_ids_2, attention_mask_2):
# 编码第一个句子
outputs_1 = self.bert(input_ids=input_ids_1, attention_mask=attention_mask_1)
pooled_output_1 = outputs_1.pooler_output
# 编码第二个句子
outputs_2 = self.bert(input_ids=input_ids_2, attention_mask=attention_mask_2)
pooled_output_2 = outputs_2.pooler_output
# 计算相似度得分
similarity_scores = torch.cosine_similarity(pooled_output_1, pooled_output_2, dim=1)
return similarity_scores
# 定义输入句子对
text_pair = [
("I love NLP.", "I hate homework."),
("This is a cat.", "This is a dog.")
]
# 将句子对转换为BERT模型所需的输入格式
inputs = tokenizer(text_pair, padding=True, truncation=True, return_tensors="pt")
input_ids_1 = inputs["input_ids"][:, 0, :] # 第一个句子的输入编码
attention_mask_1 = inputs["attention_mask"][:, 0, :] # 第一个句子的注意力掩码
input_ids_2 = inputs["input_ids"][:, 1, :] # 第二个句子的输入编码
attention_mask_2 = inputs["attention_mask"][:, 1, :] # 第二个句子的注意力掩码
# 初始化句子相似度匹配模型
model = SentenceSimilarity(bert_model)
# 使用句子相似度匹配模型进行推断
similarity_scores = model(input_ids_1, attention_mask_1, input_ids_2, attention_mask_2)
print(similarity_scores)
```
模型解释和原理技术说明:
1. SimBERT是一种基于BERT模型的相似度匹配模型,用于解决句子相似度任务,例如判断两个句子的语义相似程度。
2. 在上述代码中,首先导入了PyTorch库中的`nn.Module`模块和transformers库中的BertModel和BertTokenizer模块。
3. 加载预训练的BERT模型和分词器,使用`bert-base-uncased`作为示例。
4. 定义了一个句子相似度匹配模型类,继承自`nn.Module`。该模型包含一个BERT模型和一个线性映射层。
5. 在句子相似度匹配模型类的前向传播方法中,使用BERT模型对两个输入句子进行编码,并通过线性映射层计算相似度得分。
6. 定义了输入句子对,每个句子对包含两个句子。
7. 使用BERT分词器将句子对转换为BERT模型所需的输入格式,包括输入的编码(input_ids)和注意力掩码(attention_mask)。
8. 初始化句子相似度匹配模型实例,并将预训练的BERT模型传入。
9. 使用句子相似度匹配模型进行推断,得到两个句子的相似度得分。
10. 打印相似度得分。
通过以上代码和解释,一个NLP新手可以了解到:
- SimBERT是一种基于BERT模型的相似度匹配模型,用于解决句子相似度任务。
- 在使用PyTorch实现SimBERT模型时,需要加载预训练的BERT模型和分词器,并定义自己的模型结构。
- SimBERT模型通过对输入句子进行编码,得到上下文相关的句子向量表示,并计算相似度得分。
- 使用BERT分词器将句子对转换为BERT模型所需的输入格式,包括输入的编码(input_ids)和注意力掩码(attention_mask)。
- 初始化SimBERT模型实例后,可以将输入句子对传入模型进行推断,得到句子的相似度得分。
相关推荐
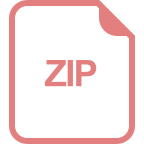
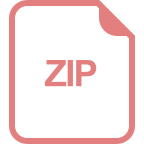
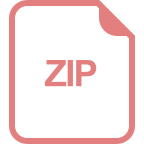
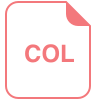
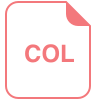
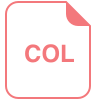
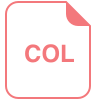
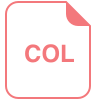









