Python matplotlib实现批量梯度下降
时间: 2023-11-19 17:06:08 浏览: 33
的过程中,如何画出损失函数随迭代次数变化的图像?
你可以使用以下代码来画出损失函数随迭代次数变化的图像:
```python
import matplotlib.pyplot as plt
# 初始化参数
theta = np.zeros([2, 1])
theta[0] = -10
theta[1] = -1
# 迭代次数和步长
iterations = 100
alpha = 0.01
# 初始化数据
X = np.random.rand(100)
Y = np.random.rand(100)
# 定义损失函数
def compute_cost(theta, X, Y):
m = len(Y)
predictions = X.dot(theta)
cost = 1/(2*m) * np.sum(np.square(predictions-Y))
return cost
# 执行梯度下降
def gradient_descent(theta, X, Y, alpha, iterations):
m = len(Y)
cost_history = np.zeros(iterations)
theta_history = np.zeros([iterations, 2])
for i in range(iterations):
predictions = X.dot(theta)
theta = theta - alpha * (1/m) * X.T.dot(predictions-Y)
theta_history[i] = theta.T
cost_history[i] = compute_cost(theta, X, Y)
return theta_history, cost_history
# 运行梯度下降并记录每次的theta和cost
theta_history, cost_history = gradient_descent(theta, X, Y, alpha, iterations)
# 画出损失函数随迭代次数变化的图像
plt.plot(range(iterations), cost_history)
plt.xlabel('迭代次数')
plt.ylabel('损失函数')
plt.show()
```
这段代码会生成一个损失函数随迭代次数变化的图像,可以清晰地看到损失函数在不断下降,说明梯度下降在有效地优化参数。
相关推荐
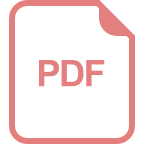
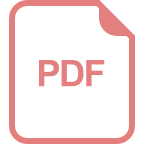














