tsp问题动态规划算法python代码
时间: 2023-07-09 12:02:11 浏览: 117
### 回答1:
TSP问题(Traveling Salesman Problem)是一种求解旅行商问题的优化算法。其目标是寻找一条路径,使得旅行商能够经过所有城市且总路径最短。以下是一种使用动态规划算法解决TSP问题的Python代码:
```python
import sys
# 动态规划函数
def tsp_dp(graph):
n = len(graph)
# 创建二维数组dp来保存子问题的解
dp = [[sys.maxsize] * n for _ in range(1 << n)]
dp[1][0] = 0
# 遍历所有的子集
for subset in range(1 << n):
# 遍历所有的城市作为终点
for end in range(n):
# 如果当前城市不在子集中,跳过
if not (subset & (1 << end)):
continue
# 遍历所有的起点城市
for start in range(n):
# 如果起点城市不在子集中或起点城市等于终点城市,跳过
if start == end or not (subset & (1 << start)):
continue
# 更新dp数组,尝试从起点到终点
dp[subset][end] = min(dp[subset][end], dp[subset ^ (1 << end)][start] + graph[start][end])
# 返回结果,即从0出发,经过所有城市后回到0的最短路径长度
return min(dp[-1][end] + graph[end][0] for end in range(1, n))
# 测试代码
if __name__ == "__main__":
graph = [
[0, 2, 9, 10],
[1, 0, 6, 4],
[15, 7, 0, 8],
[6, 3, 12, 0]
]
print(tsp_dp(graph))
```
以上代码利用动态规划的思想,通过构建一个dp数组来保存子问题的解,从而求解TSP问题。算法时间复杂度为O(n^2 * 2^n),其中n为城市的数量。
### 回答2:
以下是TSP(旅行商问题)的动态规划算法的Python代码:
```python
import sys
import math
def tsp_dp(graph, start):
num_cities = len(graph)
Visited = (1 << num_cities) - 1
dp = [[-1] * (1 << num_cities) for _ in range(num_cities)]
def tsp_helper(curr_city, visited):
if visited == Visited:
return graph[curr_city][start]
if dp[curr_city][visited] != -1:
return dp[curr_city][visited]
min_cost = sys.maxsize
for next_city in range(num_cities):
if visited & (1 << next_city) == 0:
cost = graph[curr_city][next_city] + tsp_helper(next_city, visited | (1 << next_city))
min_cost = min(min_cost, cost)
dp[curr_city][visited] = min_cost
return min_cost
return tsp_helper(start, 1 << start)
# 测试代码
graph = [
[0, 10, 15, 20],
[10, 0, 35, 25],
[15, 35, 0, 30],
[20, 25, 30, 0]
]
start_city = 0
min_cost = tsp_dp(graph, start_city)
print("最小旅行成本:", min_cost)
```
以上代码使用了动态规划的思想来解决TSP问题。在代码中,我们定义了一个二维数组`dp`来保存计算过的最小旅行成本。函数`tsp_dp`则是递归地调用`tsp_helper`函数来计算最小成本。
`tsp_helper`函数使用了位运算来表示城市的访问情况。通过递归地遍历未访问的城市,计算到达每个城市的成本,并选择最小的成本进行返回。
最后,通过给定的图和起始城市,调用`tsp_dp`函数来计算出最小的旅行成本,并打印出结果。
### 回答3:
动态规划(Dynamic Programming)是一种应用于求解最优化问题的算法思想。在TSP问题(Traveling Salesman Problem,旅行商问题)中,要求一个旅行商从一个城市出发,经过所有其他城市恰好一次,最后回到出发城市,使得总旅行路径最短。
以下是TSP问题的动态规划算法的Python代码:
```python
import sys
def tsp_dp(distance, start, visited):
if len(visited) == len(distance):
return distance[start][0]
min_distance = sys.maxsize
for city in range(len(distance)):
if city not in visited:
visited.append(city)
current_distance = distance[start][city] + tsp_dp(distance, city, visited)
min_distance = min(min_distance, current_distance)
visited.remove(city)
return min_distance
# 测试代码
distance = [
[0, 10, 15, 20],
[10, 0, 35, 25],
[15, 35, 0, 30],
[20, 25, 30, 0]
]
start_city = 0
visited_cities = [start_city]
shortest_distance = tsp_dp(distance, start_city, visited_cities)
print(f"The shortest distance is: {shortest_distance}")
```
在以上代码中,我们定义了一个`tsp_dp`函数,它采用递归的方式求解TSP问题。参数`distance`表示城市之间的距离矩阵,`start`表示当前起始城市,`visited`表示已经访问的城市集合。函数里面定义了一个基准情况,即当所有城市都被访问了一遍时,返回从当前城市回到起始城市的距离。否则,我们通过遍历未访问的城市,计算从当前城市到下一个城市的距离,加上从下一个城市作为起始城市的TSP距离,并取最小值。最终递归返回最小旅行路径距离。
上述代码的距离矩阵是一个简单的例子,你可以根据自己的实际需求修改距离矩阵以及起始城市,来求解更复杂的TSP问题。
阅读全文
相关推荐
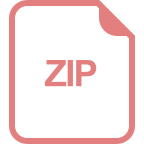


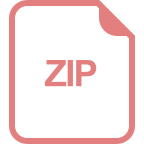
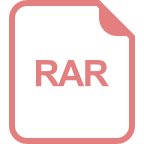
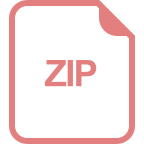
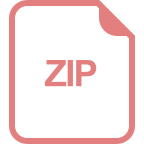
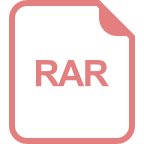
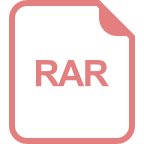
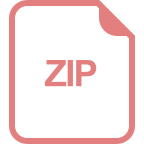
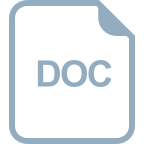




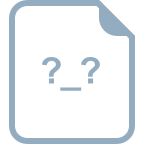