问题描述】 写一个统计文件中单词和字符个数的程序。在此,单词指的是仅由字母组成的串。诸如is7years这样的串按照两个单词计算。 【输入形式】 读取当前目录下文件in.txt,以统计该文件中单词和字符个数。 【输出形式】 输出输入文件中单词和字符个数,中间以一个空格分隔。 【样例输入】 in.txt的内容为: I am an (BeiHang)student.【样例输出】 5 25【样例说明】 该输入文件中共有5个单词(即I, am, an, BeiHang, student)及25个字符。C语言
时间: 2024-02-25 14:58:10 浏览: 65
以下是 C++ 的实现代码,可以读取当前目录下的 in.txt 文件并统计单词数和字符数:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
ifstream fin("in.txt");
string word;
int wordCount = 0, charCount = 0;
while (fin >> word) {
bool isWord = true;
for (int i = 0; i < word.size(); i++) {
if (!isalpha(word[i])) {
isWord = false;
break;
}
}
if (isWord) {
wordCount++;
}
charCount += word.size();
}
cout << wordCount << " " << charCount << endl;
return 0;
}
```
首先打开文件流 `fin`,然后通过循环读取文件中的每个单词。对于每个单词,我们判断其中是否有非字母字符,如果有,则不计入单词数。最后将单词数和字符数输出即可。
相关问题
写一个c++程序统计文件中单词和字符个数的程序。在此,单词指的是仅由字母组成的串
下面是一个简单的C++程序,用于统计文件中单词和字符的个数:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <cctype>
using namespace std;
int main()
{
string filename;
cout << "Enter filename: ";
cin >> filename;
ifstream file(filename);
if (!file.is_open()) {
cout << "Failed to open file!\n";
return 1;
}
string word;
int word_count = 0;
int char_count = 0;
while (file >> word) {
bool is_word = true;
for (char c : word) {
if (!isalpha(c)) {
is_word = false;
break;
}
}
if (is_word) {
word_count++;
}
char_count += word.length();
}
cout << "Word count: " << word_count << endl;
cout << "Character count: " << char_count << endl;
return 0;
}
```
该程序首先提示用户输入文件名,然后打开文件并检查是否成功。接下来,它循环读取文件中的每个单词,并检查它是否仅包含字母。如果是,则增加单词计数器。无论单词是否符合条件,程序都会增加字符计数器。最后,程序输出单词计数器和字符计数器的值。
请注意,该程序只考虑由字母组成的单词。如果文件中包含数字、标点符号或其他非字母字符,这些字符也会被计入字符计数器中。如果您需要一个更精确的统计程序,请根据您的需求进行修改。
用python写一个统计文件中单词和字符个数的程序。在此,单词指的是仅由字母组成的串。诸如is7years这样的串按照两个单词计算。
可以使用python中的正则表达式库re来实现。
以下是一个示例程序:
```python
import re
filename = input("请输入文件名:")
word_count = 0
char_count = 0
with open(filename, 'r') as f:
for line in f:
# 使用正则表达式匹配单词
words = re.findall(r'\b[a-zA-Z]+\b', line)
word_count += len(words)
char_count += len(line)
print("单词数:", word_count)
print("字符数:", char_count)
```
程序通过输入文件名来读取文件内容,使用正则表达式 `\b[a-zA-Z]+\b` 匹配所有仅由字母组成的单词,统计单词数和字符数并输出。注意,程序中字符数包括了文件中所有的字符,包括空格、标点符号等。如果需要排除这些字符,可以在统计字符数时进行相应的处理。
相关推荐
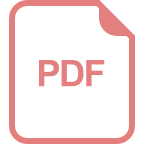
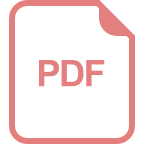
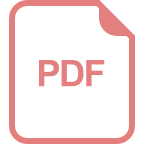













