使用Javacv合并视频和音频的stream流并将正在合并中的结果以HTTP的方式实时返回给前端
时间: 2024-06-07 16:12:02 浏览: 13
首先,您需要使用Javacv中的FFmpegFrameRecorder类来合并视频和音频流。以下是一个基本示例代码:
```java
FFmpegFrameRecorder recorder = new FFmpegFrameRecorder("output.mp4", videoWidth, videoHeight, audioChannels);
recorder.setInterleaved(true);
recorder.setVideoOption("preset", "ultrafast"); // or "medium", "slow", etc.
recorder.setVideoCodec(avcodec.AV_CODEC_ID_H264);
recorder.setAudioCodec(avcodec.AV_CODEC_ID_AAC);
recorder.setFormat("mp4");
recorder.start();
// Loop through frames and audio samples and write them to the recorder
while (/* loop condition */) {
recorder.record(frame);
recorder.recordSamples(samples);
}
recorder.stop();
```
在上面的示例中,您需要将videoWidth,videoHeight和audioChannels替换为实际值,并在while循环中提供适当的帧和音频样本。
接下来,您需要使用Java中的HttpURLConnection来将结果流实时返回给前端。以下是示例代码:
```java
URL url = new URL("http://yourserver.com/yourendpoint");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setDoOutput(true);
OutputStream outputStream = connection.getOutputStream();
FFmpegFrameRecorder recorder = new FFmpegFrameRecorder(outputStream, videoWidth, videoHeight, audioChannels);
recorder.setInterleaved(true);
recorder.setVideoOption("preset", "ultrafast"); // or "medium", "slow", etc.
recorder.setVideoCodec(avcodec.AV_CODEC_ID_H264);
recorder.setAudioCodec(avcodec.AV_CODEC_ID_AAC);
recorder.setFormat("mp4");
recorder.start();
// Loop through frames and audio samples and write them to the recorder
while (/* loop condition */) {
recorder.record(frame);
recorder.recordSamples(samples);
outputStream.flush(); // flush output stream to send data to client
}
recorder.stop();
outputStream.close();
// Get response code from server
int responseCode = connection.getResponseCode();
```
在上面的示例中,您需要将url替换为实际的HTTP端点,并在while循环中提供适当的帧和音频样本。
请注意,该示例代码仅用于演示目的。在实际情况下,您需要进行更多的错误处理和异常处理,并确保在循环结束时正确关闭所有资源。
相关推荐
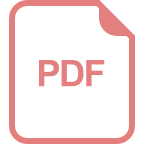
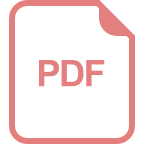














