在windows环境下用c语言编写睡眠理发师问题要求可以从键盘输入理发师和顾客数量
时间: 2024-02-27 09:59:11 浏览: 17
好的,我们可以使用Windows API中的信号量来实现进程之间的同步。
首先,定义三个信号量:chair,barber和customer。chair表示等待的椅子数量,barber表示理发师是否在工作,customer表示等待的顾客数量。
初始化chair的值为n,barber和customer的值为0。
然后,我们可以使用CreateProcess函数创建两个进程:理发师进程和顾客进程。通过使用GetStdHandle函数获取标准输入,从键盘输入理发师和顾客数量。
理发师进程的代码如下:
```c
HANDLE chair, barber, customer;
// 初始化三个信号量
chair = CreateSemaphore(NULL, n, n, NULL);
barber = CreateSemaphore(NULL, 0, 1, NULL);
customer = CreateSemaphore(NULL, 0, 1, NULL);
while (true) {
WaitForSingleObject(customer, INFINITE); // 等待顾客
WaitForSingleObject(chair, INFINITE); // 占用一把等待椅子
ReleaseSemaphore(barber, 1, NULL); // 叫醒理发师
// 理发师理发
ReleaseSemaphore(chair, 1, NULL); // 释放等待椅子
}
```
顾客进程的代码如下:
```c
HANDLE chair, barber, customer;
int num_barbers, num_customers;
// 初始化三个信号量
chair = CreateSemaphore(NULL, n, n, NULL);
barber = CreateSemaphore(NULL, 0, 1, NULL);
customer = CreateSemaphore(NULL, 0, 1, NULL);
while (true) {
WaitForSingleObject(chair, INFINITE); // 占用一把等待椅子
if (WaitForSingleObject(barber, 0) == WAIT_OBJECT_0) { // 理发师在睡觉
ReleaseSemaphore(customer, 1, NULL); // 叫醒理发师
WaitForSingleObject(barber, INFINITE); // 等待理发师准备好
// 理发师理发
ReleaseSemaphore(chair, 1, NULL); // 释放等待椅子
} else { // 理发师在工作
ReleaseSemaphore(chair, 1, NULL); // 释放等待椅子
}
}
```
完整代码如下:
```c
#include <stdio.h>
#include <Windows.h>
#define MAX_CUSTOMERS 1000
int main() {
HANDLE chair, barber, customer;
int num_barbers, num_customers;
printf("请输入理发师数量:");
scanf_s("%d", &num_barbers);
printf("请输入顾客数量:");
scanf_s("%d", &num_customers);
if (num_barbers < 1 || num_customers < 1 || num_customers > MAX_CUSTOMERS) {
printf("输入有误!\n");
return 1;
}
// 初始化三个信号量
chair = CreateSemaphore(NULL, num_customers, num_customers, NULL);
barber = CreateSemaphore(NULL, 0, num_barbers, NULL);
customer = CreateSemaphore(NULL, 0, num_customers, NULL);
// 创建理发师进程
for (int i = 0; i < num_barbers; i++) {
STARTUPINFO si;
PROCESS_INFORMATION pi;
ZeroMemory(&si, sizeof(si));
si.cb = sizeof(si);
ZeroMemory(&pi, sizeof(pi));
TCHAR cmdLine[] = TEXT("barber.exe");
if (!CreateProcess(NULL, cmdLine, NULL, NULL, FALSE, 0, NULL, NULL, &si, &pi)) {
printf("创建进程失败!\n");
return 1;
}
}
// 创建顾客进程
for (int i = 0; i < num_customers; i++) {
STARTUPINFO si;
PROCESS_INFORMATION pi;
ZeroMemory(&si, sizeof(si));
si.cb = sizeof(si);
ZeroMemory(&pi, sizeof(pi));
TCHAR cmdLine[] = TEXT("customer.exe");
if (!CreateProcess(NULL, cmdLine, NULL, NULL, FALSE, 0, NULL, NULL, &si, &pi)) {
printf("创建进程失败!\n");
return 1;
}
}
// 等待进程结束
WaitForSingleObject(GetCurrentProcess(), INFINITE);
// 关闭信号量句柄
CloseHandle(chair);
CloseHandle(barber);
CloseHandle(customer);
return 0;
}
```
理发师进程的代码:
```c
#include <Windows.h>
int main() {
HANDLE chair, barber, customer;
// 打开三个信号量
chair = OpenSemaphore(SEMAPHORE_ALL_ACCESS, FALSE, NULL);
barber = OpenSemaphore(SEMAPHORE_ALL_ACCESS, FALSE, NULL);
customer = OpenSemaphore(SEMAPHORE_ALL_ACCESS, FALSE, NULL);
while (true) {
WaitForSingleObject(customer, INFINITE); // 等待顾客
WaitForSingleObject(chair, INFINITE); // 占用一把等待椅子
ReleaseSemaphore(barber, 1, NULL); // 叫醒理发师
// 理发师理发
ReleaseSemaphore(chair, 1, NULL); // 释放等待椅子
}
// 关闭信号量句柄
CloseHandle(chair);
CloseHandle(barber);
CloseHandle(customer);
return 0;
}
```
顾客进程的代码:
```c
#include <Windows.h>
int main() {
HANDLE chair, barber, customer;
// 打开三个信号量
chair = OpenSemaphore(SEMAPHORE_ALL_ACCESS, FALSE, NULL);
barber = OpenSemaphore(SEMAPHORE_ALL_ACCESS, FALSE, NULL);
customer = OpenSemaphore(SEMAPHORE_ALL_ACCESS, FALSE, NULL);
while (true) {
WaitForSingleObject(chair, INFINITE); // 占用一把等待椅子
if (WaitForSingleObject(barber, 0) == WAIT_OBJECT_0) { // 理发师在睡觉
ReleaseSemaphore(customer, 1, NULL); // 叫醒理发师
WaitForSingleObject(barber, INFINITE); // 等待理发师准备好
// 理发师理发
ReleaseSemaphore(chair, 1, NULL); // 释放等待椅子
} else { // 理发师在工作
ReleaseSemaphore(chair, 1, NULL); // 释放等待椅子
}
}
// 关闭信号量句柄
CloseHandle(chair);
CloseHandle(barber);
CloseHandle(customer);
return 0;
}
```
相关推荐
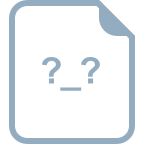
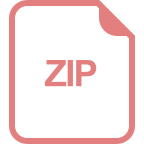














