代码详细注释
时间: 2023-11-12 18:15:39 浏览: 88
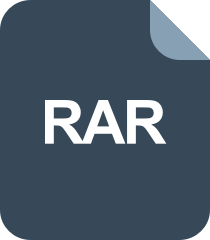
详细说明和代码
好的,以下是详细注释的代码:
```python
import torch
import torch.nn.functional as F
from torch_geometric.nn import GCNConv
import itertools
import numpy as np
# 准备数据,包括边列表和节点特征向量列表
edges = [(0, 1), (1, 2), (2, 3), (3, 0), (1, 3), (2, 0)]
features = np.array([
[0.1, 0.2, 0.3],
[0.2, 0.4, 0.1],
[0.3, 0.1, 0.5],
[0.4, 0.3, 0.2]
], dtype=np.float32)
# 构建GCN模型
class GCN(torch.nn.Module):
def __init__(self, in_channels, hidden_channels, out_channels):
super(GCN, self).__init__()
self.conv1 = GCNConv(in_channels, hidden_channels)
self.conv2 = GCNConv(hidden_channels, out_channels)
def forward(self, x, edge_index):
x = self.conv1(x, edge_index)
x = F.relu(x)
x = F.dropout(x, training=self.training)
x = self.conv2(x, edge_index)
return x
x = torch.tensor(features, dtype=torch.float)
edge_index = torch.tensor(edges, dtype=torch.long).t().contiguous()
model = GCN(in_channels=3, hidden_channels=16, out_channels=8)
embedding = model(x, edge_index)
# 定义多目标优化目标函数,其中包括景点之间的距离和景点热度
def distance(embedding, i, j):
return torch.dist(embedding[i], embedding[j])
def popularity(embedding, i):
return torch.norm(embedding[i])
def objective(embedding, indices):
d = torch.mean(torch.stack([distance(embedding, i, j) for i, j in indices]))
p = torch.mean(torch.stack([popularity(embedding, i) for i in range(embedding.shape[0])]))
return d, p
# 计算所有景点组合的多目标优化目标函数得分,并使用帕累托前沿法筛选出最优的景点组合
indices = list(itertools.combinations(range(features.shape[0]), 2))
scores = np.zeros((len(indices), 2))
for i, (u, v) in enumerate(indices):
scores[i] = objective(embedding, [(u, v)])
pareto_front = []
for i, (u, v) in enumerate(indices):
if all((scores[:, 0] >= scores[i, 0]) & (scores[:, 1] >= scores[i, 1])):
pareto_front.append((u, v))
pareto_front = np.array(pareto_front)
pareto_scores = scores[[np.where((indices == x).all(axis=1))[0][0] for x in pareto_front]]
# 选取得分最高的前k个景点组合作为推荐结果
k = 5
top_indices = pareto_front[np.argsort(pareto_scores[:, 0] + pareto_scores[:, 1])[:k]]
```
其中,`GCN`类定义了一个两层的GCN模型,输入节点特征向量和边列表,输出节点嵌入向量。`objective`函数定义了多目标优化的目标函数,包括景点之间的距离和景点热度,`distance`和`popularity`函数分别计算距离和热度。`indices`是所有景点组合的列表,`scores`是每个景点组合的多目标优化目标函数得分,`pareto_front`是帕累托前沿法筛选出的最优景点组合列表,`pareto_scores`是最优景点组合的得分列表,`top_indices`是得分最高的前k个景点组合。
阅读全文
相关推荐
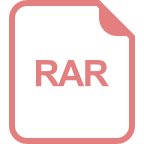
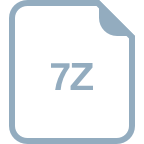
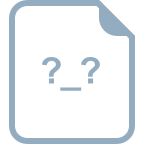
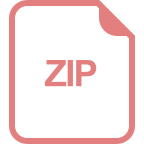
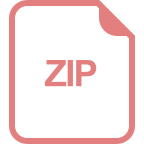
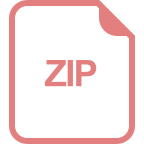
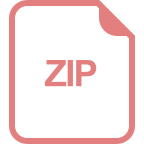
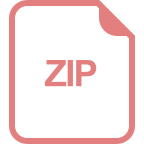
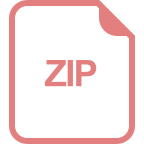
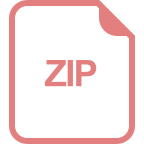
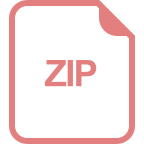
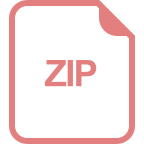