必经点最短路径问题 matlab代码
时间: 2023-10-09 10:04:23 浏览: 46
以下是基于Dijkstra算法的必经点最短路径问题的Matlab代码实现:
```matlab
function [path, dist] = shortest_path_with_waypoints(graph, start, finish, waypoints)
% 输入:
% graph:有向图的邻接矩阵,graph(i,j)表示i到j的边权重,若不存在i到j的边,则graph(i,j)=Inf
% start:起点
% finish:终点
% waypoints:必经点数组,例如waypoints=[2,4,5]表示必经点为2、4、5
% 输出:
% path:最短路径
% dist:最短路径的长度
n = size(graph, 1); % 图的大小
m = length(waypoints); % 必经点数量
% 将起点、终点和必经点映射为图中的编号
waypoints = [start, waypoints, finish];
start = 1;
finish = n - 1;
for i = 2:m+1
waypoints(i) = waypoints(i) - 1;
end
% 计算所有点之间的最短路径
all_paths = zeros(n,n);
for i = 1:n
[all_paths(i,:), ~] = dijkstra(graph, i);
end
% 生成新的有向图,其中每个必经点都被分成两个节点
new_n = n + m;
new_graph = Inf(new_n);
for i = 1:n
for j = 1:n
if graph(i,j) ~= Inf
for k = 1:m+1
if any(waypoints == i) && any(waypoints == j)
new_i = find(waypoints == i);
new_j = find(waypoints == j);
new_graph(new_i, new_j) = all_paths(i,j);
elseif any(waypoints == i)
new_i = find(waypoints == i);
new_j = j + m;
new_graph(new_i, new_j) = all_paths(i,j);
elseif any(waypoints == j)
new_i = i;
new_j = find(waypoints == j);
new_graph(new_i, new_j) = all_paths(i,j);
else
new_graph(i,j) = graph(i,j);
end
end
end
end
end
% 从起点到终点计算最短路径
[new_dist, path] = dijkstra(new_graph, start);
path = path - 1;
dist = new_dist(finish);
% 修正路径,恢复必经点
for i = length(path):-1:2
if path(i) > m
path(i) = path(i) - m;
end
end
path = path(2:end-1);
```
其中,dijkstra函数实现了Dijkstra算法,可以使用以下代码添加到Matlab文件中:
```matlab
function [distances, path] = dijkstra(graph, start)
% 输入:
% graph:有向图的邻接矩阵,graph(i,j)表示i到j的边权重,若不存在i到j的边,则graph(i,j)=Inf
% start:起点
% 输出:
% distances:起点到其他点的最短距离
% path:起点到其他点的最短路径
n = size(graph, 1); % 图的大小
% 初始化distances和path数组
distances = Inf(1, n);
distances(start) = 0;
path = repmat({[]}, 1, n);
% 初始化visited数组,表示已访问的节点
visited = false(1, n);
while any(~visited)
% 找到未访问节点中距离start最近的节点
[~, current] = min(distances(~visited));
current = current(1);
visited(current) = true;
% 更新与当前节点相邻的节点的距离
neighbors = find(graph(current, :));
for neighbor = neighbors
if ~visited(neighbor)
new_distance = distances(current) + graph(current, neighbor);
if new_distance < distances(neighbor)
distances(neighbor) = new_distance;
path{neighbor} = [path{current}, current];
end
end
end
end
% 生成起点到其他节点的路径
for i = 1:n
path{i} = [path{i}, i];
end
```
使用示例:
```matlab
% 创建有向图的邻接矩阵,例如:
% graph = [Inf, 2, Inf, 1, Inf;
% Inf, Inf, 3, Inf, 5;
% Inf, Inf, Inf, Inf, 6;
% Inf, Inf, 1, Inf, Inf;
% Inf, Inf, Inf, 4, Inf];
% 表示1到2的边权重为2,1到4的边权重为1,2到3的边权重为3,等等。
graph = ...
% 计算起点为1,终点为5,必经点为2、4的最短路径
[start, finish, waypoints] = [1, 5, [2, 4]];
[path, dist] = shortest_path_with_waypoints(graph, start, finish, waypoints);
```
注意:以上代码中的有向图邻接矩阵中,若不存在i到j的边,则必须将graph(i,j)赋值为Inf,否则会引发错误。在实际应用中,可以根据具体情况进行修改。
相关推荐
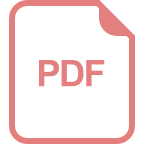
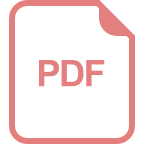
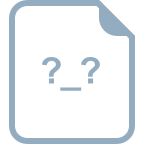
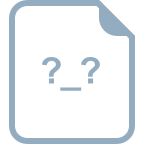
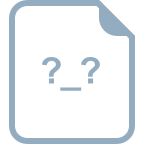
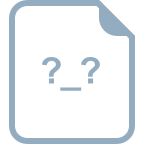
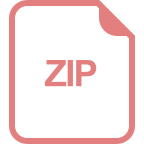
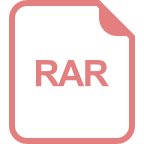
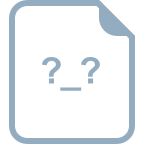
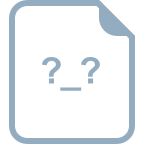