用html写一个烟花代码
时间: 2023-09-08 15:05:05 浏览: 212
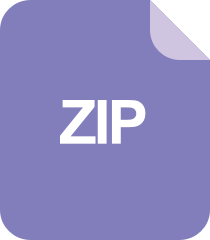
使用HTML 实现一个烟花效果
### 回答1:
好的,以下是一个简单的HTML烟花代码示例:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>烟花代码</title>
<style>
body {
background-color: black;
}
.firework {
position: absolute;
width: 5px;
height: 5px;
background-color: white;
border-radius: 50%;
animation: explode 1s linear forwards;
}
@keyframes explode {
from {
transform: scale(1);
}
to {
transform: scale(20);
opacity: 0;
}
}
</style>
</head>
<body>
<script>
function createFirework() {
const firework = document.createElement('div');
firework.className = 'firework';
firework.style.left = Math.random() * window.innerWidth + 'px';
firework.style.top = Math.random() * window.innerHeight + 'px';
document.body.appendChild(firework);
setTimeout(() => {
firework.remove();
}, 1000);
}
setInterval(() => {
createFirework();
}, 500);
</script>
</body>
</html>
```
这个代码会在页面上创建一个黑色的背景,并且每隔500毫秒就会在页面的随机位置创建一个白色的小圆点,当这个小圆点被创建时,它会放大到一个随机大小,然后逐渐消失。
### 回答2:
要用HTML编写一个烟花代码,可以使用HTML的画布和JavaScript来实现。下面是一个简单的实例:
在HTML文件中,我们首先需要创建一个画布(canvas)元素,然后定义一个用于绘制的JavaScript函数。代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>烟花特效</title>
<style>
body {
margin: 0;
padding: 0;
overflow: hidden;
}
canvas {
background-color: #000;
}
</style>
</head>
<body>
<canvas id="fireworksCanvas"></canvas>
<script>
// 定义烟花绘制函数
function drawFireworks() {
var canvas = document.getElementById("fireworksCanvas");
var ctx = canvas.getContext("2d");
var fireworks = [];
function Firework() {
this.x = Math.random() * canvas.width;
this.y = canvas.height;
this.velocityX = Math.random() * 6 - 3;
this.velocityY = Math.random() * 3 + 2;
this.alpha = 1;
this.color = getRandomColor();
}
function getRandomColor() {
var colors = [
"#ff0000",
"#00ff00",
"#0000ff",
"#ffff00",
"#ff00ff",
"#00ffff"
];
return colors[Math.floor(Math.random() * colors.length)];
}
Firework.prototype.update = function() {
this.x += this.velocityX;
this.y -= this.velocityY;
this.velocityY -= 0.1;
this.alpha -= 0.005;
};
Firework.prototype.draw = function() {
ctx.globalAlpha = this.alpha;
ctx.fillStyle = this.color;
ctx.beginPath();
ctx.arc(this.x, this.y, 3, 0, Math.PI * 2);
ctx.closePath();
ctx.fill();
};
function createFirework() {
var firework = new Firework();
fireworks.push(firework);
}
function updateFireworks() {
fireworks.forEach(function(firework, index) {
firework.update();
firework.draw();
if (firework.alpha <= 0) {
fireworks.splice(index, 1);
}
});
}
function animate() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
createFirework();
updateFireworks();
requestAnimationFrame(animate);
}
animate();
}
// 页面加载完成后执行烟花特效
window.onload = function() {
drawFireworks();
};
</script>
</body>
</html>
```
通过将以上代码复制到一个HTML文件中,然后在浏览器中打开该文件,即可看到烟花特效的效果。烟花将随机绽放出不同的颜色,并以动画方式从底部上升,最终逐渐消失。
### 回答3:
要用HTML写一个烟花代码,你可以通过使用CSS和JavaScript来制作一个简单的烟花效果。以下是一个简单的实现方式:
首先,在HTML文件中创建一个画布元素,设置宽度和高度。例如:
<canvas id="fireworksCanvas" width="800" height="600"></canvas>
接下来,使用CSS样式来设置画布的背景颜色和其他样式。例如:
<style>
#fireworksCanvas {
background-color: black;
}
</style>
然后,编写JavaScript代码来绘制烟花。你可以使用Canvas的绘图API来实现。以下是一个简单的烟花绘制函数:
<script>
function drawFirework(x, y) {
const canvas = document.getElementById('fireworksCanvas');
const ctx = canvas.getContext('2d');
// 绘制烟花的代码
}
</script>
在绘制烟花的函数中,你可以使用ctx对象来设置绘图的样式和属性,然后使用其方法来绘制形状和路径。例如,你可以使用arc方法绘制一个圆形烟花,并使用fill方法填充颜色。
绘制一个圆形烟花的代码示例:
ctx.beginPath();
ctx.arc(x, y, radius, 0, Math.PI * 2);
ctx.fillStyle = 'red';
ctx.fill();
你可以在绘制完成后调用这个函数,传入一个烟花的位置(x和y坐标)。如果你希望创建多个烟花,可以在适当的时机调用这个函数多次。
最后,你可以在你的HTML文件中使用JavaScript代码来调用绘制函数,从而触发烟花效果的显示。例如,在鼠标点击事件中调用drawFirework函数:
<script>
document.addEventListener('click', function (event) {
const x = event.clientX;
const y = event.clientY;
drawFirework(x, y);
});
</script>
这样,当用户在页面上点击时,会在点击位置绘制一个烟花。
以上是一个简单的用HTML实现烟花代码的方式,你可以根据需求和创造力进行修改和扩展。
阅读全文
相关推荐
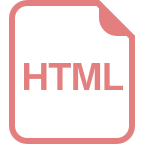














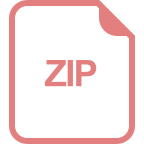