基于操作系统内核驱动,C语言代码设计实现一个简单防火墙系统的步骤
时间: 2024-03-08 07:45:50 浏览: 24
好的,下面是一个基于操作系统内核驱动的简单防火墙系统的设计实现步骤,采用C语言编写:
1. 首先需要定义一个内核模块,并在模块加载时初始化防火墙规则集合。
```c
#include <linux/module.h>
#include <linux/kernel.h>
#include <linux/netfilter.h>
#include <linux/netfilter_ipv4.h>
MODULE_LICENSE("GPL");
// 定义防火墙规则结构体
struct firewall_rule {
__be32 src_ip; // 源IP地址
__be32 dst_ip; // 目标IP地址
__be16 src_port; // 源端口号
__be16 dst_port; // 目标端口号
bool action; // 允许通过为true,拦截为false
};
// 定义防火墙规则集合
static struct firewall_rule rules[100];
static int rule_count = 0;
// 内核模块初始化函数
static int __init firewall_init(void)
{
// 初始化防火墙规则集合
// TODO: 添加默认规则
return 0;
}
// 内核模块退出函数
static void __exit firewall_exit(void)
{
// TODO: 清理资源
}
module_init(firewall_init);
module_exit(firewall_exit);
```
2. 接着需要定义一个数据包处理函数,在该函数中对拦截的数据包进行分析和处理,根据防火墙规则集合中的规则进行过滤和阻断。
```c
// 定义数据包处理函数
static unsigned int firewall_hook(unsigned int hooknum,
struct sk_buff *skb,
const struct net_device *in,
const struct net_device *out,
int (*okfn)(struct sk_buff *))
{
struct iphdr *iph = ip_hdr(skb);
struct tcphdr *tcph = tcp_hdr(skb);
// 过滤规则
for (int i = 0; i < rule_count; i++) {
if (rules[i].src_ip != 0 && iph->saddr != rules[i].src_ip)
continue;
if (rules[i].dst_ip != 0 && iph->daddr != rules[i].dst_ip)
continue;
if (rules[i].src_port != 0 && tcph->source != rules[i].src_port)
continue;
if (rules[i].dst_port != 0 && tcph->dest != rules[i].dst_port)
continue;
// 拦截或允许通过
if (!rules[i].action) {
printk(KERN_INFO "firewall: packet dropped\n");
return NF_DROP;
} else {
printk(KERN_INFO "firewall: packet allowed\n");
return NF_ACCEPT;
}
}
// 没有匹配的规则,默认允许通过
return NF_ACCEPT;
}
```
3. 接下来需要注册数据包处理函数到 netfilter 框架中。
```c
// 定义 netfilter 钩子
static struct nf_hook_ops firewall_ops = {
.hook = firewall_hook,
.pf = PF_INET,
.priority = NF_IP_PRI_FIRST,
.hooknum = NF_INET_PRE_ROUTING,
};
// 内核模块初始化函数
static int __init firewall_init(void)
{
// 初始化防火墙规则集合
// TODO: 添加默认规则
// 注册 netfilter 钩子
nf_register_hook(&firewall_ops);
return 0;
}
// 内核模块退出函数
static void __exit firewall_exit(void)
{
// 注销 netfilter 钩子
nf_unregister_hook(&firewall_ops);
// TODO: 清理资源
}
```
4. 最后需要实现防火墙规则的加载和管理功能,例如添加规则、删除规则等。
```c
// 添加防火墙规则
static void add_rule(struct firewall_rule rule)
{
if (rule_count < 100) {
rules[rule_count++] = rule;
} else {
printk(KERN_WARNING "firewall: rule table full\n");
}
}
// 删除防火墙规则
static void del_rule(int index)
{
if (index >=0 && index < rule_count) {
for (int i = index; i < rule_count - 1; i++) {
rules[i] = rules[i + 1];
}
rule_count--;
} else {
printk(KERN_WARNING "firewall: invalid rule index\n");
}
}
```
这样一个简单的基于操作系统内核驱动的防火墙系统就实现了,当然还有许多细节需要处理并且需要进行测试和调试,确保防火墙系统的稳定性和可靠性。
相关推荐
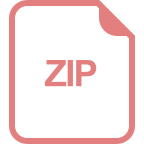
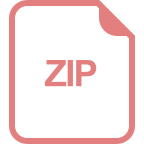














