kaggle中Contradictory, My Dear Watson这个项目怎么用pytorch实现
时间: 2023-12-06 18:39:52 浏览: 48
Contradictory, My Dear Watson是一个自然语言推断任务,可以使用PyTorch实现。下面是一个简单的实现示例:
1. 数据预处理
首先需要将训练数据和测试数据转换为PyTorch的张量,可以使用torchtext库来快速实现。具体步骤如下:
```python
import torch
from torchtext.datasets import MultiNLI
from torchtext.data import Field, LabelField, TabularDataset, BucketIterator
# 定义数据字段
text_field = Field(tokenize='spacy', tokenizer_language='en_core_web_sm', include_lengths=True)
label_field = LabelField()
# 加载数据集
train, val, test = MultiNLI.splits(text_field=text_field, label_field=label_field, root='./data')
# 构建词汇表
text_field.build_vocab(train, val, test)
# 构建数据迭代器
train_iter, val_iter, test_iter = BucketIterator.splits(
(train, val, test),
batch_sizes=(32, 32, 32),
sort_key=lambda x: len(x.premise),
sort_within_batch=True,
repeat=False
)
```
2. 定义模型
在PyTorch中,可以使用nn.Module类来定义模型。Contradictory, My Dear Watson任务可以使用Bert模型来实现。可以使用Hugging Face提供的transformers库来加载预训练的Bert模型。具体步骤如下:
```python
import torch.nn as nn
from transformers import BertModel
class BertClassifier(nn.Module):
def __init__(self, bert_model, num_labels):
super(BertClassifier, self).__init__()
self.bert = BertModel.from_pretrained(bert_model)
self.dropout = nn.Dropout(0.1)
self.linear = nn.Linear(self.bert.config.hidden_size, num_labels)
def forward(self, input_ids, attention_mask, token_type_ids):
_, pooled_output = self.bert(input_ids=input_ids, attention_mask=attention_mask, token_type_ids=token_type_ids)
dropout_output = self.dropout(pooled_output)
logits = self.linear(dropout_output)
return logits
```
3. 定义损失函数和优化器
在PyTorch中,可以使用交叉熵损失函数来计算模型的损失。可以使用torch.optim库来定义优化器。具体步骤如下:
```python
import torch.optim as optim
# 定义损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=2e-5)
```
4. 训练模型
使用定义好的模型、损失函数和优化器,以及数据迭代器,可以开始训练模型。具体步骤如下:
```python
# 训练模型
for epoch in range(5):
running_loss = 0.0
for i, batch in enumerate(train_iter):
input_ids, input_lengths = batch.premise
attention_mask = (input_ids != 1).type(torch.uint8)
token_type_ids = torch.zeros_like(input_ids)
labels = batch.label
optimizer.zero_grad()
logits = model(input_ids, attention_mask, token_type_ids)
loss = criterion(logits, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
if i % 100 == 99:
print(f'Epoch {epoch+1}, Batch {i+1}: Loss = {running_loss/100:.4f}')
running_loss = 0.0
```
5. 测试模型
训练完成后,使用测试集来评估模型的性能。具体步骤如下:
```python
# 测试模型
model.eval()
correct = 0
total = 0
with torch.no_grad():
for batch in test_iter:
input_ids, input_lengths = batch.premise
attention_mask = (input_ids != 1).type(torch.uint8)
token_type_ids = torch.zeros_like(input_ids)
labels = batch.label
logits = model(input_ids, attention_mask, token_type_ids)
_, predicted = torch.max(logits.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print(f'Test Accuracy: {correct/total:.4f}')
```
相关推荐
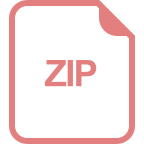
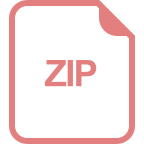














