利用CryptoAPI加/解密文件
时间: 2023-08-06 08:02:54 浏览: 46
CryptoAPI是Windows平台上的一种加密API,可以用于加密和解密文件。下面是一个简单的示例,演示如何使用CryptoAPI加密和解密文件:
加密文件:
```c++
#include <windows.h>
#include <wincrypt.h>
#include <stdio.h>
#pragma comment(lib, "crypt32.lib")
void EncryptFile(LPCWSTR lpszSourceFile, LPCWSTR lpszDestinationFile, LPCWSTR lpszPassword)
{
HCRYPTPROV hProv = NULL;
HCRYPTKEY hKey = NULL;
HCRYPTHASH hHash = NULL;
DWORD dwLen;
DWORD dwCount;
BYTE* pbBuffer = NULL;
HANDLE hSourceFile = INVALID_HANDLE_VALUE;
HANDLE hDestinationFile = INVALID_HANDLE_VALUE;
// 打开源文件
hSourceFile = CreateFile(
lpszSourceFile,
GENERIC_READ,
FILE_SHARE_READ,
NULL,
OPEN_EXISTING,
FILE_ATTRIBUTE_NORMAL,
NULL
);
if (hSourceFile == INVALID_HANDLE_VALUE)
{
printf("Error: cannot open source file.\n");
goto Exit;
}
// 创建目标文件
hDestinationFile = CreateFile(
lpszDestinationFile,
GENERIC_WRITE,
0,
NULL,
CREATE_ALWAYS,
FILE_ATTRIBUTE_NORMAL,
NULL
);
if (hDestinationFile == INVALID_HANDLE_VALUE)
{
printf("Error: cannot create destination file.\n");
goto Exit;
}
// 获取密码哈希
if (!CryptCreateHash(
hProv,
CALG_MD5,
0,
0,
&hHash))
{
printf("Error: cannot create hash.\n");
goto Exit;
}
if (!CryptHashData(
hHash,
(BYTE*)lpszPassword,
(DWORD)wcslen(lpszPassword) * sizeof(WCHAR),
0))
{
printf("Error: cannot hash password.\n");
goto Exit;
}
// 获取加密密钥
if (!CryptDeriveKey(
hProv,
CALG_RC4,
hHash,
0,
&hKey))
{
printf("Error: cannot derive key.\n");
goto Exit;
}
// 加密数据
dwLen = GetFileSize(hSourceFile, NULL);
pbBuffer = new BYTE[dwLen];
if (!ReadFile(hSourceFile, pbBuffer, dwLen, &dwCount, NULL))
{
printf("Error: cannot read source file.\n");
goto Exit;
}
if (!CryptEncrypt(
hKey,
NULL,
TRUE,
0,
pbBuffer,
&dwLen,
dwLen))
{
printf("Error: cannot encrypt data.\n");
goto Exit;
}
// 写入加密后的数据到目标文件
if (!WriteFile(hDestinationFile, pbBuffer, dwLen, &dwCount, NULL))
{
printf("Error: cannot write destination file.\n");
goto Exit;
}
Exit:
if (hSourceFile != INVALID_HANDLE_VALUE)
{
CloseHandle(hSourceFile);
}
if (hDestinationFile != INVALID_HANDLE_VALUE)
{
CloseHandle(hDestinationFile);
}
if (hHash != NULL)
{
CryptDestroyHash(hHash);
}
if (hKey != NULL)
{
CryptDestroyKey(hKey);
}
if (hProv != NULL)
{
CryptReleaseContext(hProv, 0);
}
if (pbBuffer != NULL)
{
delete[] pbBuffer;
}
}
```
解密文件:
```c++
#include <windows.h>
#include <wincrypt.h>
#include <stdio.h>
#pragma comment(lib, "crypt32.lib")
void DecryptFile(LPCWSTR lpszSourceFile, LPCWSTR lpszDestinationFile, LPCWSTR lpszPassword)
{
HCRYPTPROV hProv = NULL;
HCRYPTKEY hKey = NULL;
HCRYPTHASH hHash = NULL;
DWORD dwLen;
DWORD dwCount;
BYTE* pbBuffer = NULL;
HANDLE hSourceFile = INVALID_HANDLE_VALUE;
HANDLE hDestinationFile = INVALID_HANDLE_VALUE;
// 打开源文件
hSourceFile = CreateFile(
lpszSourceFile,
GENERIC_READ,
FILE_SHARE_READ,
NULL,
OPEN_EXISTING,
FILE_ATTRIBUTE_NORMAL,
NULL
);
if (hSourceFile == INVALID_HANDLE_VALUE)
{
printf("Error: cannot open source file.\n");
goto Exit;
}
// 创建目标文件
hDestinationFile = CreateFile(
lpszDestinationFile,
GENERIC_WRITE,
0,
NULL,
CREATE_ALWAYS,
FILE_ATTRIBUTE_NORMAL,
NULL
);
if (hDestinationFile == INVALID_HANDLE_VALUE)
{
printf("Error: cannot create destination file.\n");
goto Exit;
}
// 获取密码哈希
if (!CryptCreateHash(
hProv,
CALG_MD5,
0,
0,
&hHash))
{
printf("Error: cannot create hash.\n");
goto Exit;
}
if (!CryptHashData(
hHash,
(BYTE*)lpszPassword,
(DWORD)wcslen(lpszPassword) * sizeof(WCHAR),
0))
{
printf("Error: cannot hash password.\n");
goto Exit;
}
// 获取解密密钥
if (!CryptDeriveKey(
hProv,
CALG_RC4,
hHash,
0,
&hKey))
{
printf("Error: cannot derive key.\n");
goto Exit;
}
// 解密数据
dwLen = GetFileSize(hSourceFile, NULL);
pbBuffer = new BYTE[dwLen];
if (!ReadFile(hSourceFile, pbBuffer, dwLen, &dwCount, NULL))
{
printf("Error: cannot read source file.\n");
goto Exit;
}
if (!CryptDecrypt(
hKey,
NULL,
TRUE,
0,
pbBuffer,
&dwLen))
{
printf("Error: cannot decrypt data.\n");
goto Exit;
}
// 写入解密后的数据到目标文件
if (!WriteFile(hDestinationFile, pbBuffer, dwLen, &dwCount, NULL))
{
printf("Error: cannot write destination file.\n");
goto Exit;
}
Exit:
if (hSourceFile != INVALID_HANDLE_VALUE)
{
CloseHandle(hSourceFile);
}
if (hDestinationFile != INVALID_HANDLE_VALUE)
{
CloseHandle(hDestinationFile);
}
if (hHash != NULL)
{
CryptDestroyHash(hHash);
}
if (hKey != NULL)
{
CryptDestroyKey(hKey);
}
if (hProv != NULL)
{
CryptReleaseContext(hProv, 0);
}
if (pbBuffer != NULL)
{
delete[] pbBuffer;
}
}
```
要使用这些功能,只需调用EncryptFile和DecryptFile函数,并传递源文件路径、目标文件路径和加密/解密密码即可。请注意,这只是一个简单的示例,实际应用中需要考虑更多的安全问题。
相关推荐
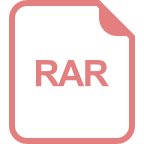
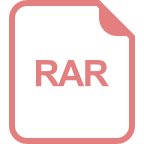








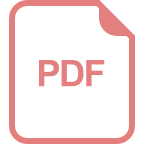
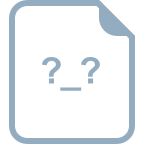