写一个Android 第一屏:包含标题写为“考试系统”;包含用户及密码输入框及登录按钮。 要求:限制用户名必须是固定10位数字,密码必须为6位数字,点击登录,若用户名为:2023062200,密码为:111111,跳转第二屏;否则提示用户名密码错误。的文档
时间: 2023-07-16 16:17:20 浏览: 82
这里提供一个简单的Android应用程序示例,其中包含一个登录页面和一个第二屏页面。用户必须输入正确的用户名和密码才能登录到第二屏页面。
1. 在Android Studio中创建一个新项目,选择Empty Activity模板。
2. 在res/layout目录下创建一个名为activity_login.xml的新布局文件,用于定义登录页面的UI元素。
```
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp">
<TextView
android:id="@+id/title_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="考试系统"
android:textSize="24sp"
android:textStyle="bold" />
<EditText
android:id="@+id/user_edit"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/title_text"
android:layout_marginTop="32dp"
android:hint="请输入10位数字的用户名"
android:inputType="number"
android:maxLength="10" />
<EditText
android:id="@+id/password_edit"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/user_edit"
android:layout_marginTop="16dp"
android:hint="请输入6位数字的密码"
android:inputType="number"
android:maxLength="6" />
<Button
android:id="@+id/login_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/password_edit"
android:layout_marginTop="32dp"
android:text="登录" />
</RelativeLayout>
```
3. 在res/layout目录下创建一个名为activity_second.xml的新布局文件,用于定义第二屏页面的UI元素。
```
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="欢迎来到第二屏页面!"
android:textSize="24sp"
android:textStyle="bold" />
</LinearLayout>
```
4. 在MainActivity.java中添加以下代码,用于处理登录按钮的点击事件并验证用户名和密码是否正确。
```
public class MainActivity extends AppCompatActivity {
private EditText mUserEdit;
private EditText mPasswordEdit;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
mUserEdit = findViewById(R.id.user_edit);
mPasswordEdit = findViewById(R.id.password_edit);
Button loginButton = findViewById(R.id.login_button);
loginButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String user = mUserEdit.getText().toString();
String password = mPasswordEdit.getText().toString();
if (user.equals("2023062200") && password.equals("111111")) {
Intent intent = new Intent(MainActivity.this, SecondActivity.class);
startActivity(intent);
} else {
Toast.makeText(MainActivity.this, "用户名或密码错误!", Toast.LENGTH_SHORT).show();
}
}
});
}
}
```
5. 在SecondActivity.java中添加以下代码,用于显示第二屏页面。
```
public class SecondActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
}
}
```
6. 运行应用程序,并在登录页面输入正确的用户名和密码。如果验证通过,应用程序将跳转到第二屏页面。如果验证失败,应用程序将显示一个错误提示。
注意:此示例应用程序的安全性不够强大,仅供学习和演示目的。在实际应用程序中,需要使用更安全的身份验证方法来保护用户数据。
阅读全文
相关推荐
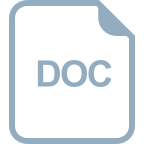
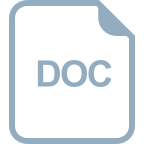
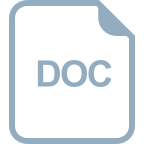




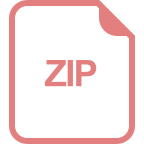



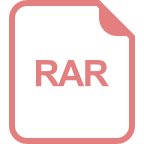
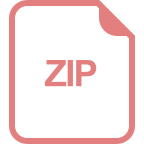
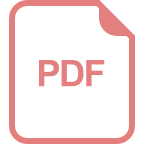


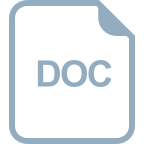
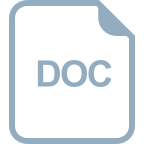