使用C#语言在visual studio 平台上写一段CAD二次开发.net代码用于生产类库dll文件,CAD加载dll文件后,须由用户在CAD界面选定2根直线,输入2根直线等分数量N,计算直线N等分后N+1个等分点坐标,并由2条直线等分点成对生成N+1条直线,直线需显示在CAD界面
时间: 2024-03-31 07:34:49 浏览: 57
好的,根据您的要求,我可以给您提供一段C#代码,用于实现CAD二次开发.net,生成类库dll文件,实现选定2根直线,输入等分数量N,计算直线N等分后N+1个等分点坐标,并由2条直线等分点成对生成N+1条直线,直线在CAD界面上显示的功能。代码如下:
```csharp
// 引入AutoCAD COM组件
using Autodesk.AutoCAD.Runtime;
using Autodesk.AutoCAD.ApplicationServices;
using Autodesk.AutoCAD.DatabaseServices;
using Autodesk.AutoCAD.Geometry;
namespace MyCADPlugin
{
public class MyCommands
{
[CommandMethod("MyLineDivide")]
public static void LineDivide()
{
// 获取当前文档和数据库
Document doc = Application.DocumentManager.MdiActiveDocument;
Database db = doc.Database;
// 选择第一条直线
PromptEntityOptions peo1 = new PromptEntityOptions("请选择第一根直线:");
peo1.SetRejectMessage("请选择直线!");
peo1.AddAllowedClass(typeof(Line), true);
PromptEntityResult per1 = doc.Editor.GetEntity(peo1);
if (per1.Status != PromptStatus.OK) return;
ObjectId lineId1 = per1.ObjectId;
// 选择第二条直线
PromptEntityOptions peo2 = new PromptEntityOptions("请选择第二根直线:");
peo2.SetRejectMessage("请选择直线!");
peo2.AddAllowedClass(typeof(Line), true);
PromptEntityResult per2 = doc.Editor.GetEntity(peo2);
if (per2.Status != PromptStatus.OK) return;
ObjectId lineId2 = per2.ObjectId;
// 输入等分数量
PromptIntegerOptions pio = new PromptIntegerOptions("请输入等分数量:");
pio.AllowNegative = false;
pio.AllowZero = false;
PromptIntegerResult pir = doc.Editor.GetInteger(pio);
if (pir.Status != PromptStatus.OK) return;
int divideNum = pir.Value;
// 获取直线1和直线2的起点和终点坐标
Point3d startPoint1, endPoint1, startPoint2, endPoint2;
using (Transaction trans = db.TransactionManager.StartTransaction())
{
Line line1 = trans.GetObject(lineId1, OpenMode.ForRead) as Line;
startPoint1 = line1.StartPoint;
endPoint1 = line1.EndPoint;
Line line2 = trans.GetObject(lineId2, OpenMode.ForRead) as Line;
startPoint2 = line2.StartPoint;
endPoint2 = line2.EndPoint;
trans.Commit();
}
// 计算等分点坐标
Point3d[] dividePoints1 = new Point3d[divideNum + 1];
Point3d[] dividePoints2 = new Point3d[divideNum + 1];
for (int i = 0; i <= divideNum; i++)
{
dividePoints1[i] = startPoint1 + (endPoint1 - startPoint1) * i / divideNum;
dividePoints2[i] = startPoint2 + (endPoint2 - startPoint2) * i / divideNum;
}
// 生成等分线段
using (Transaction trans = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)trans.GetObject(db.BlockTableId, OpenMode.ForRead);
BlockTableRecord btr = (BlockTableRecord)trans.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForWrite);
for (int i = 0; i <= divideNum; i++)
{
Line newLine = new Line(dividePoints1[i], dividePoints2[i]);
btr.AppendEntity(newLine);
trans.AddNewlyCreatedDBObject(newLine, true);
}
trans.Commit();
}
// 刷新CAD界面
doc.Editor.Regen();
}
}
}
```
这段代码实现了一个名为"MyCommands"的类,其中包含一个名为"MyLineDivide"的方法,用于实现选定2根直线,输入等分数量N,计算直线N等分后N+1个等分点坐标,并由2条直线等分点成对生成N+1条直线,直线在CAD界面上显示的功能。该方法通过AutoCAD COM组件获取当前文档和数据库,然后通过用户交互方式获取两条直线和等分数量,计算等分点坐标,生成等分线段,最后刷新CAD界面,使直线显示出来。注意,该代码需要在Visual Studio中编译成类库dll文件,并在AutoCAD中加载使用。
阅读全文
相关推荐
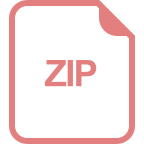
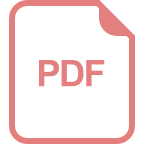
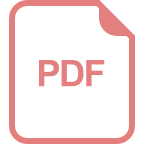














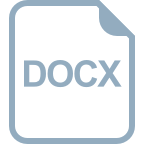
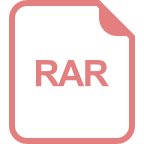