计一个学生类,该学生类主要属性有:学号、姓名和语文、数学、英语、物理、化学、历史、地理、生物八门课程的成绩,可以考虑八门课程成绩用一个列表存储,最后删除张三的语文成绩,修改数学成绩,然后输出
时间: 2023-10-28 17:01:22 浏览: 51
可以按照以下方式定义一个学生类:
```python
class Student:
def __init__(self, student_id, name, scores):
self.student_id = student_id
self.name = name
self.scores = scores
def remove_score(self, subject):
if subject in self.scores:
self.scores.pop(subject)
def update_score(self, subject, score):
if subject in self.scores:
self.scores[subject] = score
def print_info(self):
print(f"学号:{self.student_id}")
print(f"姓名:{self.name}")
print("各科成绩:")
for subject, score in self.scores.items():
print(f"{subject}:{score}")
```
其中,`__init__` 方法初始化学生对象的属性,接收三个参数:学号、姓名和一个字典,该字典用于存储各科成绩,其中键为科目名称,值为分数。
`remove_score` 方法用于删除某一科目的成绩,接收一个参数:要删除的科目名称。
`update_score` 方法用于修改某一科目的成绩,接收两个参数:要修改的科目名称和新的分数。
`print_info` 方法用于输出学生的信息,包括学号、姓名和各科成绩。
现在我们可以创建一个学生对象,并进行删除和修改操作:
```python
# 创建一个学生对象
scores = {"语文": 89, "数学": 92, "英语": 88, "物理": 85, "化学": 90, "历史": 87, "地理": 83, "生物": 91}
student = Student("001", "张三", scores)
# 删除语文成绩
student.remove_score("语文")
# 修改数学成绩
student.update_score("数学", 95)
# 输出学生信息
student.print_info()
```
输出结果如下:
```
学号:001
姓名:张三
各科成绩:
数学:95
英语:88
物理:85
化学:90
历史:87
地理:83
生物:91
```
相关推荐
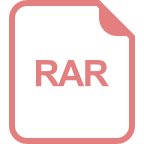
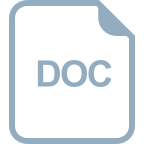
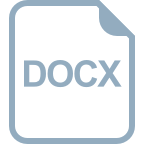















