c++,查找某个值是否在set容器内
时间: 2024-02-17 12:06:24 浏览: 32
以下是示例代码:
```c++
#include <set>
int main() {
std::set<int> my_set = {1, 2, 3, 4, 5}; // 假设 set 容器内已经存在元素 1,2,3,4,5
// 查找值为 3 是否在 set 容器内
const int value = 3;
const bool is_found = my_set.find(value) != my_set.end();
// 输出查找结果
if (is_found) {
std::cout << "值 " << value << " 存在于 set 容器内" << std::endl;
} else {
std::cout << "值 " << value << " 不存在于 set 容器内" << std::endl;
}
return 0;
}
```
代码中的 `find` 方法返回指向查找到的元素的迭代器,如果未找到则返回 `end` 迭代器。因此,通过判断 `find` 方法的返回值是否等于 `end` 迭代器,可以确定元素是否存在于 set 容器内。
相关问题
c++判断set中是否有某个值
在 C++ 的 set 容器中,要判断是否存在某个值,可以使用 find 函数来查找。find 函数会在 set 中查找指定元素,如果找到了,返回指向该元素的迭代器;如果没有找到,返回 set 的 end 迭代器。
例如,下面的代码演示了如何使用 find 函数来查找 set 中是否存在某个值:
```c++
#include <set>
#include <iostream>
int main()
{
std::set<int> s = { 1, 2, 3, 4, 5 };
int x = 3;
auto it = s.find(x);
if (it != s.end())
{
std::cout << "Found " << x << " in set" << std::endl;
}
else
{
std::cout << x << " not found in set" << std::endl;
}
return 0;
}
```
在上面的代码中,我们首先创建了一个 set 对象,并使用 find 函数查找 set 中是否存在值为 3 的元素。由于 set 中存在值为 3 的元素,因此 find 函数返回指向该元素的迭代器,if 语句输出 Found 3 in set。
如果要判断 set 中是否存在某个值,也可以使用 count 函数。count 函数会返回 set 中等于指定值的元素个数,因此如果元素个数大于 0,就表示 set 中存在指定值。例如,下面的代码演示了如何使用 count 函数来判断 set 中是否存在某个值:
```c++
#include <set>
#include <iostream>
int main()
{
std::set<int> s = { 1, 2, 3, 4, 5 };
int x = 3;
if (s.count(x) > 0)
{
std::cout << "Found " << x << " in set" << std::endl;
}
else
{
std::cout << x << " not found in set" << std::endl;
}
return 0;
}
```
在上面的代码中,我们使用 count 函数来判断 set 中是否存在值为 3 的元素。由于 set 中存在值为 3 的元素,因此 count 函数返回值大于 0,if 语句输出 Found 3 in set。
c++ set容器
C++中的set是一个关联容器,它可以存储一组不重复的元素,并且按照一定的顺序进行排序。set容器中的元素默认是按照升序排列的,也可以自定义排序规则。set容器的底层实现是使用红黑树来实现的,因此其插入、删除、查找等操作的时间复杂度均为O(logn)。
在使用set容器时,需要包含头文件<set>,并使用set模板类定义一个set对象。例如:
```c++
#include <set>
using namespace std;
set<int> mySet;
```
以上代码定义了一个存储整型数据的set容器对象mySet。接下来就可以通过调用set容器的成员函数来对mySet进行操作了。
相关推荐
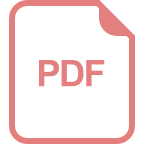
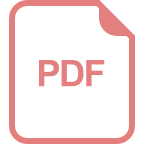
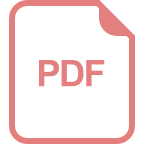












