用python代码写一个五子棋
时间: 2023-09-05 09:04:46 浏览: 82
### 回答1:
好的,下面是使用Python语言编写五子棋游戏的代码:
```python
import numpy as np
# 定义棋盘大小和棋子种类
BOARD_SIZE = 15
EMPTY, BLACK, WHITE = 0, 1, 2
class Board:
"""定义一个五子棋棋盘"""
def __init__(self):
self.board = np.zeros((BOARD_SIZE, BOARD_SIZE), dtype=np.int32)
self.current_player = BLACK # 当前落子方
def move(self, row, col):
"""在(row, col)位置落子"""
if self.board[row][col] == EMPTY:
self.board[row][col] = self.current_player
self.current_player = WHITE if self.current_player == BLACK else BLACK
def check(self, player):
"""检查是否胜利"""
# 检查横向是否有五个连续的棋子
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE - 4):
if np.all(self.board[i][j:j+5] == player):
return True
# 检查纵向是否有五个连续的棋子
for i in range(BOARD_SIZE - 4):
for j in range(BOARD_SIZE):
if np.all(self.board[i:i+5, j] == player):
return True
# 检查正斜向是否有五个连续的棋子
for i in range(BOARD_SIZE - 4):
for j in range(BOARD_SIZE - 4):
if np.all(self.board[i:i+5, j:j+5].diagonal() == player):
return True
# 检查反斜向是否有五个连续的棋子
for i in range(4, BOARD_SIZE):
for j in range(BOARD_SIZE - 4):
if np.all(np.fliplr(self.board)[i:i-5, j:j+5].diagonal() == player):
return True
return False
def is_full(self):
"""判断棋盘是否已经满了"""
return np.all(self.board != EMPTY)
def get_board(self):
"""返回当前的棋盘"""
return self.board
def get_current_player(self):
"""返回当前的落子方"""
return self.current_player
```
以上代码实现了一个五子棋的棋盘类,可以在其中调用move方法落子,check方法判断是否胜利,is_full方法判断棋盘是否已经满了。
### 回答2:
五子棋是一种非常经典的棋类游戏,下面是一个用Python代码实现的五子棋游戏:
```python
# 定义棋盘大小
BOARD_SIZE = 15
# 初始化棋盘
def initBoard():
board = []
for i in range(BOARD_SIZE):
row = ['+'] * BOARD_SIZE
board.append(row)
return board
# 打印棋盘
def printBoard(board):
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
print(board[i][j], end=' ')
print()
# 判断胜利条件
def isWin(board, x, y):
dirs = [(1, 0), (0, 1), (1, 1), (1, -1)]
for dir in dirs:
count = 1
dx, dy = dir
# 向前搜索
while count < 5:
if x+count*dx >= 0 and x+count*dx < BOARD_SIZE and y+count*dy >= 0 and y+count*dy < BOARD_SIZE:
if board[x+count*dx][y+count*dy] == board[x][y]:
count += 1
else:
break
else:
break
# 判断是否五子连珠
if count == 5:
return True
return False
# 下棋
def play(board):
turn = 1
while True:
printBoard(board)
if turn % 2 == 1:
print("轮到黑方下棋:")
player = 'X'
else:
print("轮到白方下棋:")
player = 'O'
x = int(input("请输入横坐标(0-14):"))
y = int(input("请输入纵坐标(0-14):"))
if x >= 0 and x < BOARD_SIZE and y >= 0 and y < BOARD_SIZE and board[x][y] == '+':
board[x][y] = player
if isWin(board, x, y):
print("恭喜", player, "方获胜!")
break
turn += 1
# 主函数
def main():
board = initBoard()
play(board)
main()
```
运行该代码,可以玩五子棋游戏。黑方使用X表示,白方使用O表示。按照提示,交替输入横纵坐标,就可以在棋盘上下棋。当有一方连成五子时,游戏结束,输出获胜方。
### 回答3:
五子棋是一种对弈棋类游戏,通过下棋的方式,在棋盘上连成五个相同颜色的棋子即为胜利。下面是一个使用Python编写的简化版五子棋的示例代码:
```python
# 定义棋盘的大小(15行15列)
SIZE = 15
# 定义两种棋子的标记:玩家和电脑
PLAYER = 'X'
COMPUTER = 'O'
# 创建棋盘
def create_board():
board = [[' ' for _ in range(SIZE)] for _ in range(SIZE)]
return board
# 打印棋盘
def print_board(board):
print(' ' + ' '.join([str(i) for i in range(SIZE)]))
for i in range(SIZE):
print(str(i) + ' ' + ' '.join(board[i]))
# 判断是否获胜
def check_win(board, row, col, player):
directions = [(1, 0), (0, 1), (1, 1), (1, -1)] # 横、竖、左斜、右斜
for dx, dy in directions:
count = 1
x, y = row, col
while count < 5:
x += dx
y += dy
if x < 0 or x >= SIZE or y < 0 or y >= SIZE or board[x][y] != player:
break
count += 1
x, y = row, col
while count < 5:
x -= dx
y -= dy
if x < 0 or x >= SIZE or y < 0 or y >= SIZE or board[x][y] != player:
break
count += 1
if count >= 5:
return True
return False
# 主函数
def main():
board = create_board()
print_board(board)
player_turn = True # 玩家先手
while True:
if player_turn:
print('轮到玩家下棋:')
row = int(input('请输入行号: '))
col = int(input('请输入列号: '))
if board[row][col] == ' ':
board[row][col] = PLAYER
player_turn = False
else:
print('该位置已经有棋子,请重新输入。')
else:
print('电脑下棋中...')
# 简化版的电脑移动策略,随机选择一个空位置
empty_positions = []
for i in range(SIZE):
for j in range(SIZE):
if board[i][j] == ' ':
empty_positions.append((i, j))
position = random.choice(empty_positions)
board[position[0]][position[1]] = COMPUTER
player_turn = True
print_board(board)
if check_win(board, row, col, PLAYER):
print('玩家获胜!')
break
elif check_win(board, position[0], position[1], COMPUTER):
print('电脑获胜!')
break
if __name__ == "__main__":
main()
```
这个代码是一个简化版的五子棋游戏,使用了随机选择空位置的电脑移动策略。玩家可以通过输入行号和列号来下棋,电脑则根据随机选取的空位置来下棋。游戏会根据每一步的情况打印当前的棋盘,并通过判断是否获胜来结束游戏。
相关推荐
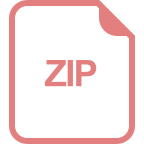
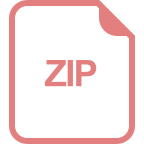









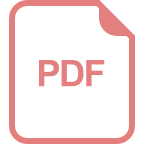
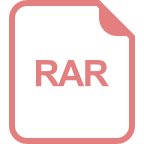
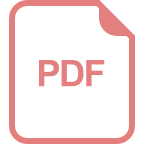
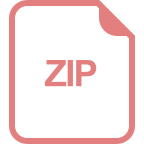