文字转语音,使用java实现,写出具体java代码
时间: 2023-09-27 10:05:28 浏览: 127
### 回答1:
我可以回答这个问题。以下是使用Java实现文字转语音的示例代码:
import java.io.*;
import javax.sound.sampled.*;
public class TextToSpeech {
public static void main(String[] args) {
try {
// Set up the audio format
AudioFormat format = new AudioFormat(800, 8, 1, true, true);
// Get a line to the speakers
DataLine.Info info = new DataLine.Info(SourceDataLine.class, format);
SourceDataLine speakers = (SourceDataLine) AudioSystem.getLine(info);
speakers.open(format);
speakers.start();
// Get the text to speak
String text = "你好,我是CSDN开发的AI语言模型。";
// Create a synthesizer
SpeechSynthesizer synthesizer = Central.createSynthesizer(null);
synthesizer.allocate();
// Set the synthesizer properties
synthesizer.getSynthesizerProperties().setVoice("cmn-Hans-CN");
synthesizer.getSynthesizerProperties().setSpeakingRate(150);
// Synthesize the text to an audio stream
ByteArrayOutputStream out = new ByteArrayOutputStream();
synthesizer.synthesize(text, out);
out.close();
// Play the audio stream through the speakers
byte[] audio = out.toByteArray();
speakers.write(audio, , audio.length);
speakers.drain();
speakers.close();
// Deallocate the synthesizer
synthesizer.deallocate();
} catch (Exception e) {
e.printStackTrace();
}
}
}
### 回答2:
文字转语音是一种将文字转化为可听的语音输出的技术。在Java中实现文字转语音可以使用Java的Speech API(JSAPI)来实现。下面是一个简单的Java代码示例:
首先,需要导入相关的类和接口:
```java
import javax.speech.*;
import javax.speech.synthesis.*;
import java.util.Locale;
```
接下来,需要创建一个SpeechSynthesizer对象,并设置输出模式和语言环境:
```java
SpeechSynthesizer synthesizer = Central.createSynthesizer(null);
synthesizer.allocate();
synthesizer.getSynthesizerProperties().setOutputToDefaultAudioDevice();
synthesizer.getSynthesizerProperties().setVoice(new Voice(null, null, 350, 150, null));
synthesizer.resume();
```
然后,可以使用synthesize方法将文字转换成语音,并进行播放:
```java
synthesizer.speakPlainText("你好,欢迎使用文字转语音技术", null);
synthesizer.waitEngineState(Synthesizer.QUEUE_EMPTY);
synthesizer.deallocate();
```
最后,记得在代码的结尾处调用synthesize方法和deallocate方法释放资源。
以上是一个简单的实现文字转语音的Java代码示例。当然,还可以根据需求进一步定制和处理语音输出的效果。
### 回答3:
文字转语音是一种将文字信息转化为可听的语音输出的技术。Java作为一种功能强大的编程语言,可以使用第三方库来实现文字转语音的功能。
在Java中,我们可以使用FreeTTS这个开源库来实现文字转语音的功能。首先,我们需要下载FreeTTS的jar包,并在项目中引入它。
然后,我们可以编写以下Java代码来使用FreeTTS实现文字转语音的功能:
```java
import com.sun.speech.freetts.Voice;
import com.sun.speech.freetts.VoiceManager;
public class TextToSpeech {
private static final String VOICENAME = "kevin16";
public static void main(String[] args) {
String text = "文字转语音测试"; // 要转换的文字
Voice voice;
VoiceManager voiceManager = VoiceManager.getInstance();
voice = voiceManager.getVoice(VOICENAME);
if (voice != null) {
voice.allocate(); // 分配资源
voice.speak(text); // 将文字转为语音输出
voice.deallocate(); // 释放资源
} else {
System.out.println("找不到该语音库!");
}
}
}
```
在上述代码中,我们先定义了一个`VOICENAME`常量,用于指定使用的语音库。在这里,我们选择了`kevin16`作为语音库。
然后,我们在`main`方法中,创建一个VoiceManager实例,并使用`getVoice`方法获取指定名称的语音。然后,我们向语音系统分配资源,并使用`speak`方法将文字转化为语音输出。
最后,记得在使用完成后,调用`deallocate`方法释放语音资源。如果无法找到指定的语音库,会输出相应的提示信息。
需要注意的是,使用FreeTTS进行文字转语音需要将字体文件fonts.xml配置正确,且需要安装对应的语音库。
相关推荐
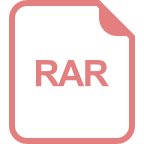
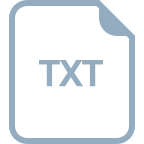
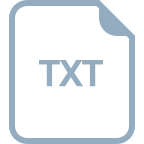








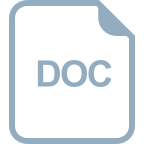
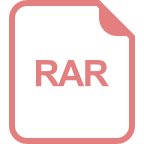
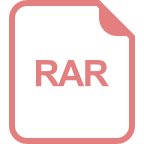
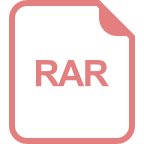
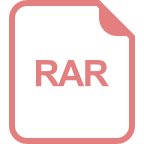
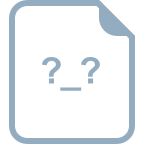