1.利用注解、反射和工厂模式设计一个简单的IoC容器。 2.该IoC容器包含3个注解和一个IoC容器类(AnnotationConfigApplicationContext),其定义如下。 注解 含义 @Component 标注Bean @Autowired 标注需要被注入的对象 @Configuration 标注为配置类 @ComponentScan 注解扫描器
时间: 2024-03-27 22:37:43 浏览: 19
好的,针对您的问题,我可以给您提供一些思路和代码实现。
首先,我们来看一下注解的定义:
1. @Component
该注解用于标注Bean,表示该类需要被IoC容器管理。
```
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
public @interface Component {
String value() default "";
}
```
2. @Autowired
该注解用于标注需要被注入的对象,表示该对象需要从IoC容器中获取。
```
@Target({ElementType.FIELD, ElementType.CONSTRUCTOR})
@Retention(RetentionPolicy.RUNTIME)
public @interface Autowired {
String value() default "";
}
```
3. @Configuration
该注解用于标注为配置类,表示该类中定义的Bean需要被IoC容器管理。
```
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
public @interface Configuration {
String value() default "";
}
```
接下来,我们来看一下IoC容器类的实现。我们可以定义一个AnnotationConfigApplicationContext类,其中包含一个Map用于存储Bean的定义和实例。
```
public class AnnotationConfigApplicationContext {
private Map<String, Object> beanMap = new HashMap<>();
public AnnotationConfigApplicationContext(Class<?> configClass) throws Exception {
// 扫描所有的Component注解,并将类定义加入到beanMap中
scanComponents(configClass);
// 处理所有的Autowired注解,并注入Bean依赖
injectDependencies();
}
// 扫描所有的Component注解
private void scanComponents(Class<?> configClass) throws Exception {
ComponentScan componentScan = configClass.getAnnotation(ComponentScan.class);
if (componentScan != null) {
String[] basePackages = componentScan.value();
for (String basePackage : basePackages) {
String packagePath = basePackage.replace(".", "/");
URL url = Thread.currentThread().getContextClassLoader().getResource(packagePath);
if (url == null) {
continue;
}
File basePackageFile = new File(url.toURI());
for (File file : basePackageFile.listFiles()) {
String fileName = file.getName();
if (fileName.endsWith(".class")) {
String className = fileName.substring(0, fileName.lastIndexOf("."));
Class<?> clazz = Class.forName(basePackage + "." + className);
Component component = clazz.getAnnotation(Component.class);
if (component != null) {
String beanName = component.value();
if (beanName.equals("")) {
beanName = className.substring(0, 1).toLowerCase() + className.substring(1);
}
Object bean = clazz.getDeclaredConstructor().newInstance();
beanMap.put(beanName, bean);
}
}
}
}
}
}
// 处理所有的Autowired注解
private void injectDependencies() throws Exception {
for (Object bean : beanMap.values()) {
for (Field field : bean.getClass().getDeclaredFields()) {
Autowired autowired = field.getAnnotation(Autowired.class);
if (autowired != null) {
String beanName = autowired.value();
if (beanName.equals("")) {
beanName = field.getType().getSimpleName().substring(0, 1).toLowerCase() + field.getType().getSimpleName().substring(1);
}
field.setAccessible(true);
field.set(bean, beanMap.get(beanName));
}
}
}
}
// 获取Bean实例
public Object getBean(String beanName) {
return beanMap.get(beanName);
}
}
```
最后,我们来看一下如何使用该IoC容器。我们可以定义一个配置类,并在该类中定义需要被IoC容器管理的Bean。
```
@Configuration
@ComponentScan("com.example")
public class AppConfig {
@Component
public class UserService {
public void sayHello() {
System.out.println("Hello, world!");
}
}
@Component
public class UserController {
@Autowired
private UserService userService;
public void sayHello() {
userService.sayHello();
}
}
}
```
然后,我们可以创建一个AnnotationConfigApplicationContext实例,并通过getBean方法获取Bean实例。
```
public static void main(String[] args) throws Exception {
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
UserController userController = (UserController) context.getBean("userController");
userController.sayHello();
}
```
相关推荐
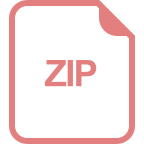
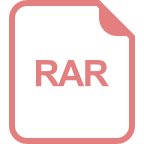














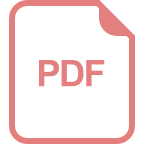