go语言编写一个学生申请借书的借书平台
时间: 2024-03-30 13:34:53 浏览: 58
好的,以下是一个简单的学生申请借书的借书平台的Go语言代码示例:
```go
package main
import (
"fmt"
"time"
)
type Book struct {
Title string
Author string
Quantity int
}
type Student struct {
Name string
StudentID string
Borrowed []string
}
var books = []Book{
{"The Great Gatsby", "F. Scott Fitzgerald", 3},
{"To Kill a Mockingbird", "Harper Lee", 2},
{"1984", "George Orwell", 5},
{"Animal Farm", "George Orwell", 1},
}
var students = make(map[string]Student)
func main() {
for {
fmt.Println("Welcome to the Book Borrowing System!")
fmt.Println("1. View available books")
fmt.Println("2. Borrow a book")
fmt.Println("3. Return a book")
fmt.Println("4. View borrowed books")
fmt.Println("5. Exit")
var choice int
fmt.Scanln(&choice)
switch choice {
case 1:
viewBooks()
case 2:
borrowBook()
case 3:
returnBook()
case 4:
viewBorrowedBooks()
case 5:
fmt.Println("Goodbye!")
return
default:
fmt.Println("Invalid choice, please try again.")
}
fmt.Println()
}
}
func viewBooks() {
fmt.Println("Available Books:")
for i, book := range books {
fmt.Printf("%d. %s by %s (%d available)\n", i+1, book.Title, book.Author, book.Quantity)
}
}
func borrowBook() {
var studentID string
fmt.Println("Please enter your student ID:")
fmt.Scanln(&studentID)
if _, ok := students[studentID]; !ok {
var studentName string
fmt.Println("Please enter your name:")
fmt.Scanln(&studentName)
students[studentID] = Student{Name: studentName, StudentID: studentID}
}
var bookIndex int
fmt.Println("Please enter the number of the book you wish to borrow:")
fmt.Scanln(&bookIndex)
if bookIndex < 1 || bookIndex > len(books) {
fmt.Println("Invalid book number, please try again.")
return
}
book := &books[bookIndex-1]
if book.Quantity == 0 {
fmt.Println("Sorry, that book is not available for borrowing.")
return
}
book.Quantity--
students[studentID].Borrowed = append(students[studentID].Borrowed, book.Title)
fmt.Printf("Book '%s' borrowed successfully by %s.\n", book.Title, students[studentID].Name)
}
func returnBook() {
var studentID string
fmt.Println("Please enter your student ID:")
fmt.Scanln(&studentID)
student, ok := students[studentID]
if !ok {
fmt.Println("Invalid student ID, please try again.")
return
}
if len(student.Borrowed) == 0 {
fmt.Println("You haven't borrowed any books.")
return
}
var bookIndex int
fmt.Println("Please enter the number of the book you wish to return:")
fmt.Scanln(&bookIndex)
if bookIndex < 1 || bookIndex > len(student.Borrowed) {
fmt.Println("Invalid book number, please try again.")
return
}
bookTitle := student.Borrowed[bookIndex-1]
for i, book := range books {
if book.Title == bookTitle {
book.Quantity++
break
}
}
students[studentID].Borrowed = append(student.Borrowed[:bookIndex-1], student.Borrowed[bookIndex:]...)
fmt.Printf("Book '%s' returned successfully by %s.\n", bookTitle, student.Name)
}
func viewBorrowedBooks() {
var studentID string
fmt.Println("Please enter your student ID:")
fmt.Scanln(&studentID)
student, ok := students[studentID]
if !ok {
fmt.Println("Invalid student ID, please try again.")
return
}
if len(student.Borrowed) == 0 {
fmt.Println("You haven't borrowed any books.")
return
}
fmt.Printf("Borrowed Books by %s:\n", student.Name)
for i, bookTitle := range student.Borrowed {
fmt.Printf("%d. %s\n", i+1, bookTitle)
}
}
```
这个示例中,我们定义了两个结构体:`Book` 和 `Student`。我们用 `Book` 结构体来表示图书,其中包含书名、作者和数量等属性。我们用 `Student` 结构体来表示学生,其中包含姓名、学号和已借阅的书籍列表。
我们还定义了两个变量:`books` 和 `students`。`books` 是一个 `Book` 类型的切片,表示所有可借阅的图书。`students` 是一个 `map[string]Student` 类型的映射,表示所有学生,其中键是学号,值是 `Student` 类型的结构体。
在 `main()` 函数中,我们实现了一个简单的命令行菜单,用于浏览可借阅的图书、借阅图书、归还图书和查看已借阅的图书。在上述函数中,我们利用 Go 语言的标准输入/输出库来实现用户输入和输出的交互。我们还使用 Go 语言的切片和映射来管理图书和学生的信息。
希望这个简单的示例可以帮助你了解如何使用 Go 语言开发一个学生申请借书的借书平台。
阅读全文
相关推荐
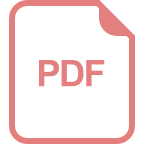
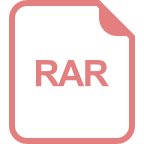















