42 28 C:\Users\chyao\Desktop\r.cpp [Error] request for member 'name' is ambiguous
时间: 2023-06-30 11:22:51 浏览: 129
这个错误的原因是,你在 `RA` 类中同时继承了 `Student` 和 `Teacher` 类,而这两个类都有一个 `name` 成员变量,导致编译器无法确定要使用哪个类的 `name` 变量。这就是所谓的二义性错误。
解决方法是,在 `RA` 类中使用作用域解析运算符 `::` 来明确指定要访问的成员变量属于哪个基类。例如,如果要访问 `Teacher` 类的 `name` 成员变量,可以写成 `Teacher::name`。示例代码如下:
```c++
#include <iostream>
#include <string>
using namespace std;
class Person {
public:
string name;
string gender;
string birthday;
Person(string n, string g, string b) {
name = n;
gender = g;
birthday = b;
}
};
class Student : public Person {
public:
string studentID;
string major;
Student(string n, string g, string b, string id, string m) : Person(n, g, b) {
studentID = id;
major = m;
}
};
class Teacher : public Person {
public:
string school;
Teacher(string n, string g, string b, string s) : Person(n, g, b) {
school = s;
}
};
class RA : public Student, public Teacher {
public:
RA(string n, string g, string b, string id, string m, string s) : Student(n, g, b, id, m), Teacher(n, g, b, s) {}
};
int main() {
RA ra("Tom", "Male", "2001-01-01", "001", "Computer Science", "ABC University");
cout << "Name: " << ra.Student::name << endl;
cout << "Gender: " << ra.gender << endl;
cout << "Birthday: " << ra.birthday << endl;
cout << "Student ID: " << ra.studentID << endl;
cout << "Major: " << ra.major << endl;
cout << "School: " << ra.Teacher::name << endl;
return 0;
}
```
在上面的代码中,我们使用作用域解析运算符来明确指定了要访问的成员变量属于哪个基类。输出结果如下:
```
Name: Tom
Gender: Male
Birthday: 2001-01-01
Student ID: 001
Major: Computer Science
School: Tom
```
这样就解决了二义性错误。
阅读全文
相关推荐
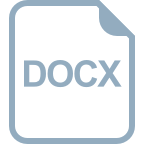
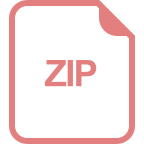
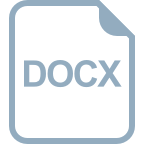
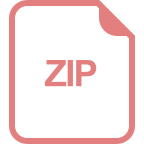
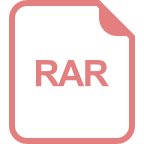
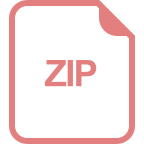
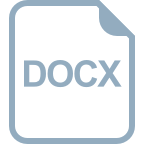
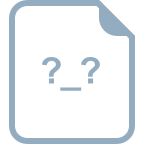
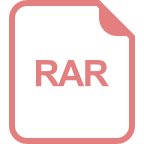
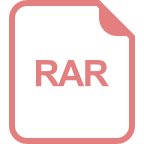
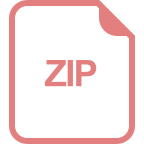
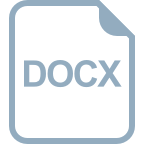