编写以下代码,使用javaswing编写一款五子棋游戏,要求有规则说明
时间: 2024-03-08 13:50:49 浏览: 59
好的,以下是一个简单的五子棋游戏示例,包括了规则说明:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class Gobang extends JFrame implements ActionListener, MouseListener {
private final int WIDTH = 600; // 窗口宽度
private final int HEIGHT = 600; // 窗口高度
private final int ROWS = 15; // 棋盘行数
private final int COLS = 15; // 棋盘列数
private final int CELL_SIZE = 40; // 棋盘格子大小
private final int MARGIN = 40; // 棋盘距离窗口边缘的距离
private final int PLAYER1 = 1; // 玩家1
private final int PLAYER2 = 2; // 玩家2
private int[][] board = new int[ROWS][COLS]; // 棋盘数组,0:空,1:玩家1,2:玩家2
private int player = PLAYER1; // 当前玩家
private JPanel boardPanel; // 棋盘面板
private JLabel statusLabel; // 状态提示标签
public Gobang() {
setTitle("五子棋");
setSize(WIDTH, HEIGHT);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setLayout(new BorderLayout());
// 创建棋盘面板
boardPanel = new JPanel() {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
drawBoard(g); // 绘制棋盘
drawPieces(g); // 绘制棋子
}
};
boardPanel.setPreferredSize(new Dimension(WIDTH, HEIGHT));
boardPanel.addMouseListener(this);
// 创建状态提示标签
statusLabel = new JLabel("玩家1,请下棋");
statusLabel.setPreferredSize(new Dimension(WIDTH, 50));
statusLabel.setHorizontalAlignment(SwingConstants.CENTER);
// 添加面板和标签到窗口
add(boardPanel, BorderLayout.CENTER);
add(statusLabel, BorderLayout.SOUTH);
setVisible(true);
}
// 绘制棋盘
private void drawBoard(Graphics g) {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
g.drawRect(MARGIN + j * CELL_SIZE, MARGIN + i * CELL_SIZE, CELL_SIZE, CELL_SIZE);
}
}
}
// 绘制棋子
private void drawPieces(Graphics g) {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] == PLAYER1) {
g.setColor(Color.BLACK);
g.fillOval(MARGIN + j * CELL_SIZE - CELL_SIZE / 2, MARGIN + i * CELL_SIZE - CELL_SIZE / 2, CELL_SIZE, CELL_SIZE);
} else if (board[i][j] == PLAYER2) {
g.setColor(Color.WHITE);
g.fillOval(MARGIN + j * CELL_SIZE - CELL_SIZE / 2, MARGIN + i * CELL_SIZE - CELL_SIZE / 2, CELL_SIZE, CELL_SIZE);
}
}
}
}
// 判断游戏是否结束
private boolean isGameOver(int row, int col) {
// 判断横向是否连成五个棋子
int count = 1;
for (int i = col - 1; i >= 0; i--) {
if (board[row][i] == board[row][col]) {
count++;
} else {
break;
}
}
for (int i = col + 1; i < COLS; i++) {
if (board[row][i] == board[row][col]) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
// 判断纵向是否连成五个棋子
count = 1;
for (int i = row - 1; i >= 0; i--) {
if (board[i][col] == board[row][col]) {
count++;
} else {
break;
}
}
for (int i = row + 1; i < ROWS; i++) {
if (board[i][col] == board[row][col]) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
// 判断左上到右下是否连成五个棋子
count = 1;
for (int i = row - 1, j = col - 1; i >= 0 && j >= 0; i--, j--) {
if (board[i][j] == board[row][col]) {
count++;
} else {
break;
}
}
for (int i = row + 1, j = col + 1; i < ROWS && j < COLS; i++, j++) {
if (board[i][j] == board[row][col]) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
// 判断左下到右上是否连成五个棋子
count = 1;
for (int i = row + 1, j = col - 1; i < ROWS && j >= 0; i++, j--) {
if (board[i][j] == board[row][col]) {
count++;
} else {
break;
}
}
for (int i = row - 1, j = col + 1; i >= 0 && j < COLS; i--, j++) {
if (board[i][j] == board[row][col]) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
// 判断是否平局
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] == 0) {
return false;
}
}
}
return true;
}
// 切换玩家
private void switchPlayer() {
if (player == PLAYER1) {
player = PLAYER2;
statusLabel.setText("玩家2,请下棋");
} else {
player = PLAYER1;
statusLabel.setText("玩家1,请下棋");
}
}
// 提示错误信息
private void showError(String message) {
JOptionPane.showMessageDialog(this, message, "错误", JOptionPane.ERROR_MESSAGE);
}
// 处理鼠标点击事件
@Override
public void mouseClicked(MouseEvent e) {
int row = (e.getY() - MARGIN + CELL_SIZE / 2) / CELL_SIZE;
int col = (e.getX() - MARGIN + CELL_SIZE / 2) / CELL_SIZE;
if (row < 0 || row >= ROWS || col < 0 || col >= COLS) {
showError("超出棋盘范围!");
return;
}
if (board[row][col] != 0) {
showError("该位置已经有棋子了!");
return;
}
board[row][col] = player;
boardPanel.repaint();
if (isGameOver(row, col)) {
if (player == PLAYER1) {
JOptionPane.showMessageDialog(this, "玩家1获胜!", "游戏结束", JOptionPane.INFORMATION_MESSAGE);
} else {
JOptionPane.showMessageDialog(this, "玩家2获胜!", "游戏结束", JOptionPane.INFORMATION_MESSAGE);
}
System.exit(0);
}
switchPlayer();
}
@Override
public void mousePressed(MouseEvent e) {}
@Override
public void mouseReleased(MouseEvent e) {}
@Override
public void mouseEntered(MouseEvent e) {}
@Override
public void mouseExited(MouseEvent e) {}
@Override
public void actionPerformed(ActionEvent e) {}
public static void main(String[] args) {
new Gobang();
}
}
```
游戏规则:
1. 两个玩家轮流下棋,黑方先手。
2. 棋盘大小为 15 行 * 15 列,棋子大小为 40 * 40 像素。
3. 当一方在横、竖、斜方向上连续下了 5 个棋子时,游戏结束,该方获胜。
4. 当棋盘被下满时,游戏结束,平局。
5. 玩家不能在已经有棋子的位置下棋。
6. 点击“关闭”按钮或按下“Esc”键可以退出游戏。
阅读全文
相关推荐
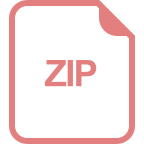
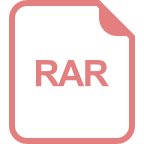

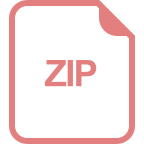
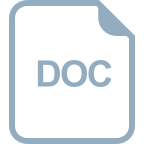
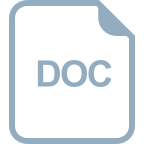
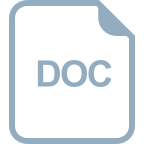
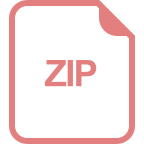
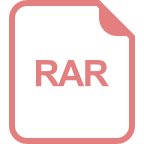
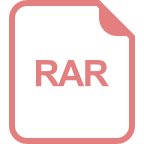
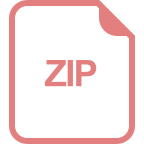
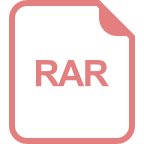
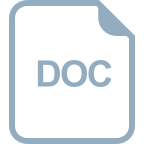
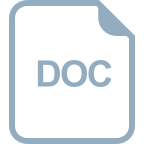
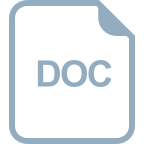
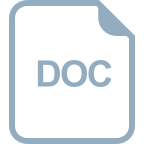
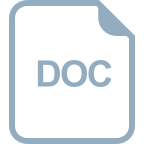