java 编程要求 在Student.java中定义类Student,其成员和方法具体要求如下。 1、所有属性都是私有成员,包括: 表示学号的int类型的id、简记为(int id); 姓名(String name), 英语成绩(double englishScore); 数学成绩(double mathScore); 计算机成绩(double computerScore); 总成绩(double totalScore)。 2、所有方法都是公开方法,包括: 无参构造方法(将所有成绩赋值为0); 两个参数的构造方法(先调用无参构造方法、再使用两个参数依次给id和name赋值); 返回学号和姓名的方法,方法名依次为:getId、getName; 返回各科成绩的方法:getEnglishScore、getMathScore、getComputerScore, 设置各科科成绩的方法、方法名分别为:setEnglishScore、setMathScore、setComputerScore,要求任何一门成绩的改变都要重新计算总成绩,且三个方法皆无返回值; 返回总成绩的方法getSum。
时间: 2023-06-27 07:04:11 浏览: 168
请参考以下代码:
```java
public class Student {
private int id;
private String name;
private double englishScore;
private double mathScore;
private double computerScore;
private double totalScore;
// 无参构造方法
public Student() {
this.id = 0;
this.name = "";
this.englishScore = 0;
this.mathScore = 0;
this.computerScore = 0;
this.totalScore = 0;
}
// 两个参数的构造方法
public Student(int id, String name) {
this();
this.id = id;
this.name = name;
}
// 获取学号
public int getId() {
return id;
}
// 获取姓名
public String getName() {
return name;
}
// 获取英语成绩
public double getEnglishScore() {
return englishScore;
}
// 获取数学成绩
public double getMathScore() {
return mathScore;
}
// 获取计算机成绩
public double getComputerScore() {
return computerScore;
}
// 设置英语成绩
public void setEnglishScore(double englishScore) {
this.englishScore = englishScore;
calculateTotalScore();
}
// 设置数学成绩
public void setMathScore(double mathScore) {
this.mathScore = mathScore;
calculateTotalScore();
}
// 设置计算机成绩
public void setComputerScore(double computerScore) {
this.computerScore = computerScore;
calculateTotalScore();
}
// 计算总成绩
private void calculateTotalScore() {
this.totalScore = this.englishScore + this.mathScore + this.computerScore;
}
// 获取总成绩
public double getSum() {
return totalScore;
}
}
```
使用示例:
```java
public class Main {
public static void main(String[] args) {
Student student = new Student(1, "张三");
System.out.println("学号:" + student.getId());
System.out.println("姓名:" + student.getName());
System.out.println("英语成绩:" + student.getEnglishScore());
System.out.println("数学成绩:" + student.getMathScore());
System.out.println("计算机成绩:" + student.getComputerScore());
System.out.println("总成绩:" + student.getSum());
student.setEnglishScore(80);
student.setMathScore(90);
student.setComputerScore(95);
System.out.println("学号:" + student.getId());
System.out.println("姓名:" + student.getName());
System.out.println("英语成绩:" + student.getEnglishScore());
System.out.println("数学成绩:" + student.getMathScore());
System.out.println("计算机成绩:" + student.getComputerScore());
System.out.println("总成绩:" + student.getSum());
}
}
```
阅读全文
相关推荐


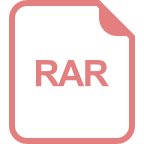
















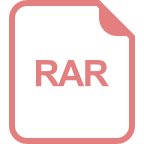