linux c 多线程
时间: 2023-07-03 14:13:15 浏览: 56
在 Linux C 中,使用多线程可以通过 pthread 库来实现。pthread 库是 POSIX 标准线程库的实现,可以跨平台运行。下面是一个简单的多线程示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 5
void *thread_func(void *arg)
{
int tid = *(int *)arg;
printf("Thread %d is running\n", tid);
pthread_exit(NULL);
}
int main()
{
pthread_t tid[NUM_THREADS];
int i, rc;
for (i = 0; i < NUM_THREADS; i++) {
printf("Creating thread %d\n", i);
rc = pthread_create(&tid[i], NULL, thread_func, &i);
if (rc) {
printf("Error: return code from pthread_create() is %d\n", rc);
exit(-1);
}
}
pthread_exit(NULL);
return 0;
}
```
上面的示例中,我们定义了一个 `thread_func` 函数,它接受一个 `void *` 类型的参数,并在该函数中输出线程的编号。在 `main` 函数中,我们使用 `pthread_create` 函数创建了 `NUM_THREADS` 个线程,并将它们的编号作为参数传递给 `thread_func` 函数。最后,我们调用 `pthread_exit` 函数等待所有线程结束。
需要注意的是,在使用多线程时,需要注意线程之间的同步和互斥,避免出现竞态条件和死锁等问题。在 Linux C 中,可以使用互斥量(mutex)和条件变量(condition variable)等机制来实现同步和互斥。
阅读全文
相关推荐














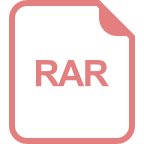
