Java spring mybatis MySQL 编写短信模板的增删改查,使用SMStemplateDTO作为实体类名称,数据库字段有seq_no,template_id,push_plan_tp_cd,template_name,template_content,creator_no,create_time,modifier_no,mod_time,opror_instno,需要使用mapper.xml,mapper,controller,servive,serviceimpl,dto,controller需要使用public restresponse并抛出异常生成错误代码,并且把controller拆分出api,错误日志使用log.true,并在impl中判断pushplantpcd是否为空,不为空则无法删除和修改
时间: 2024-02-17 15:00:47 浏览: 19
好的,让我来回答您的问题。
对于Java Spring + MyBatis + MySQL编写短信模板的增删改查,您可以按照以下步骤进行:
1. 创建SMSTemplateDTO实体类,包含seq_no,template_id,push_plan_tp_cd,template_name,template_content,creator_no,create_time,modifier_no,mod_time,opror_instno等字段。
2. 创建mapper.xml文件,编写相应的SQL语句,例如:
```
<select id="getSMSTemplateById" parameterType="int" resultType="com.example.dto.SMSTemplateDTO">
SELECT * FROM sms_template WHERE seq_no = #{id}
</select>
<insert id="insertSMSTemplate" parameterType="com.example.dto.SMSTemplateDTO">
INSERT INTO sms_template (template_id, push_plan_tp_cd, template_name, template_content, creator_no, create_time, modifier_no, mod_time, opror_instno)
VALUES (#{templateId}, #{pushPlanTpCd}, #{templateName}, #{templateContent}, #{creatorNo}, #{createTime}, #{modifierNo}, #{modTime}, #{oprorInstno})
</insert>
<update id="updateSMSTemplate" parameterType="com.example.dto.SMSTemplateDTO">
UPDATE sms_template SET push_plan_tp_cd = #{pushPlanTpCd}, template_name = #{templateName}, template_content = #{templateContent}, modifier_no = #{modifierNo}, mod_time = #{modTime}, opror_instno = #{oprorInstno}
WHERE seq_no = #{seqNo} AND push_plan_tp_cd IS NULL
</update>
<delete id="deleteSMSTemplate" parameterType="int">
DELETE FROM sms_template WHERE seq_no = #{id} AND push_plan_tp_cd IS NULL
</delete>
```
3. 创建对应的mapper接口,例如:
```
public interface SMSTemplateMapper {
SMSTemplateDTO getSMSTemplateById(int id);
void insertSMSTemplate(SMSTemplateDTO dto);
void updateSMSTemplate(SMSTemplateDTO dto);
void deleteSMSTemplate(int id);
}
```
4. 创建SMSTemplateService接口和实现类SMSTemplateServiceImpl,实现增删改查的业务逻辑。
5. 创建SMSTemplateController,包含对应的增删改查API,例如:
```
@RestController
@RequestMapping("/api/sms/template")
public class SMSTemplateController {
@Autowired
private SMSTemplateService smsTemplateService;
@GetMapping("/{id}")
public RestResponse getSMSTemplate(@PathVariable int id) {
SMSTemplateDTO dto = smsTemplateService.getSMSTemplateById(id);
if (dto == null) {
throw new NotFoundException("SMS Template not found");
}
return RestResponse.success(dto);
}
@PostMapping("/")
public RestResponse insertSMSTemplate(@RequestBody SMSTemplateDTO dto) {
smsTemplateService.insertSMSTemplate(dto);
return RestResponse.success();
}
@PutMapping("/{id}")
public RestResponse updateSMSTemplate(@PathVariable int id, @RequestBody SMSTemplateDTO dto) {
dto.setSeqNo(id);
smsTemplateService.updateSMSTemplate(dto);
return RestResponse.success();
}
@DeleteMapping("/{id}")
public RestResponse deleteSMSTemplate(@PathVariable int id) {
smsTemplateService.deleteSMSTemplate(id);
return RestResponse.success();
}
@ExceptionHandler(Exception.class)
public RestResponse handleException(Exception e) {
log.error("Error occurred: {}", e.getMessage());
return RestResponse.error("Internal Server Error");
}
}
```
6. 在SMSTemplateServiceImpl中对push_plan_tp_cd进行判断,例如:
```
public void updateSMSTemplate(SMSTemplateDTO dto) {
if (dto.getPushPlanTpCd() != null) {
throw new BadRequestException("Cannot update SMS Template with push plan");
}
smsTemplateMapper.updateSMSTemplate(dto);
}
public void deleteSMSTemplate(int id) {
SMSTemplateDTO dto = smsTemplateMapper.getSMSTemplateById(id);
if (dto == null) {
throw new NotFoundException("SMS Template not found");
}
if (dto.getPushPlanTpCd() != null) {
throw new BadRequestException("Cannot delete SMS Template with push plan");
}
smsTemplateMapper.deleteSMSTemplate(id);
}
```
7. 在SMSTemplateController中使用RestResponse和异常处理生成错误代码,并拆分出API。
以上就是Java Spring + MyBatis + MySQL编写短信模板的增删改查的步骤,希望对您有帮助。
相关推荐
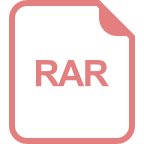















