实验七文件系统拷贝功能模拟
时间: 2023-07-19 22:07:31 浏览: 99
好的,实验七是模拟文件系统拷贝功能,我们可以通过编写一个 Python 脚本来实现。
首先,我们需要确定源文件和目标文件的路径。可以通过命令行参数传入或者手动设置。
我们可以使用 Python 的 `os` 模块来获取文件信息,并使用 `shutil` 模块来进行文件拷贝操作。
以下是一个简单的示例代码,可以实现将一个文件从源路径拷贝到目标路径的功能:
```python
import os
import shutil
# 源文件路径
src_path = "path/to/source/file"
# 目标文件路径
dst_path = "path/to/destination/file"
# 获取源文件信息
src_file_info = os.stat(src_path)
# 判断源文件是否存在
if not os.path.exists(src_path):
print("源文件不存在!")
exit()
# 判断目标文件夹是否存在,如果不存在则创建
dst_dir = os.path.dirname(dst_path)
if not os.path.exists(dst_dir):
os.makedirs(dst_dir)
# 拷贝文件
shutil.copy2(src_path, dst_path)
# 获取拷贝后的文件信息
dst_file_info = os.stat(dst_path)
# 输出拷贝前后的文件大小
print("源文件大小:", src_file_info.st_size)
print("目标文件大小:", dst_file_info.st_size)
```
在实际应用中,我们还可以加入更多的判断,例如判断目标文件是否已经存在,如果存在则需要进行覆盖等操作。
阅读全文
相关推荐


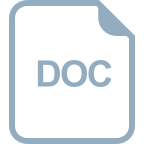






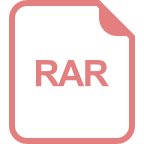





