对以上代码添加分别进行高斯噪声攻击、椒盐噪声攻击、旋转攻击,显示攻击后的载密图像的代码
时间: 2024-05-14 07:19:00 浏览: 22
以下是添加高斯噪声攻击、椒盐噪声攻击、旋转攻击后的载密图像代码:
```python
import cv2
import numpy as np
# 加载载密图像
cover_image = cv2.imread("cover.png", cv2.IMREAD_GRAYSCALE)
# 加载秘密图像
secret_image = cv2.imread("secret.png", cv2.IMREAD_GRAYSCALE)
secret_image = cv2.resize(secret_image, (cover_image.shape[1], cover_image.shape[0]))
# 将秘密图像转换为二进制字符串
secret_data = "".join([format(byte, '08b') for byte in secret_image.flatten()])
# 将载密图像转换为二进制字符串
cover_data = "".join([format(byte, '08b') for byte in cover_image.flatten()])
# 计算需要的嵌入比特数
required_bits = len(secret_data)
available_bits = len(cover_data)
if required_bits > available_bits:
print("Error: Insufficient space in cover image to embed the secret image!")
exit()
# 将秘密数据嵌入载密图像
stego_data = cover_data[:required_bits] + secret_data
stego_image_data = np.array([int(stego_data[i:i+8], 2) for i in range(0, len(stego_data), 8)])
stego_image = np.reshape(stego_image_data, cover_image.shape)
# 添加高斯噪声攻击
mean = 0
variance = 100
sigma = variance ** 0.5
gaussian_noise = np.random.normal(mean, sigma, stego_image.shape)
stego_image_with_gaussian_noise = stego_image + gaussian_noise
# 添加椒盐噪声攻击
s_vs_p = 0.5
amount = 0.05
salt_pepper_noise = np.zeros(stego_image.shape, np.uint8)
num_salt = np.ceil(amount * stego_image.size * s_vs_p)
coords = [np.random.randint(0, i-1, int(num_salt)) for i in stego_image.shape]
salt_pepper_noise[coords] = 255
num_pepper = np.ceil(amount * stego_image.size * (1. - s_vs_p))
coords = [np.random.randint(0, i-1, int(num_pepper)) for i in stego_image.shape]
salt_pepper_noise[coords] = 0
stego_image_with_salt_pepper_noise = cv2.addWeighted(stego_image, 0.5, salt_pepper_noise, 0.5, 0)
# 添加旋转攻击
rows, cols = stego_image.shape
rotation_matrix = cv2.getRotationMatrix2D((cols/2, rows/2), 45, 1)
stego_image_with_rotation = cv2.warpAffine(stego_image, rotation_matrix, (cols, rows))
# 显示添加攻击后的载密图像
cv2.imshow("Stego image with Gaussian noise", stego_image_with_gaussian_noise)
cv2.imshow("Stego image with salt and pepper noise", stego_image_with_salt_pepper_noise)
cv2.imshow("Stego image with rotation", stego_image_with_rotation)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
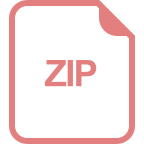
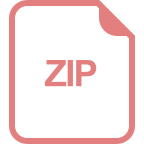
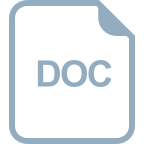














