vue2 + jsplumb 实现点击添加连线
时间: 2023-07-29 11:13:45 浏览: 226
要实现点击添加连线,首先需要安装 jsplumb 和 vue2 的相关依赖包。
然后,可以通过以下步骤实现点击添加连线的功能:
1. 在 Vue2 中创建一个画布(canvas)元素,用于绘制连线。
2. 在画布上绑定鼠标点击事件,当用户点击画布时,获取当前鼠标的位置,作为起始点。
3. 接着,绑定鼠标移动事件,当用户拖动鼠标时,获取当前鼠标的位置,作为结束点。同时,实时更新起始点和结束点之间的连线。
4. 最后,绑定鼠标释放事件,当用户释放鼠标时,将起始点和结束点之间的连线保存到数据中,以便后续使用。
下面是一个简单的实现示例代码:
```html
<template>
<div>
<div ref="canvas" @mousedown="handleMouseDown" @mousemove="handleMouseMove" @mouseup="handleMouseUp"></div>
</div>
</template>
<script>
import jsPlumb from 'jsplumb'
export default {
mounted() {
// 初始化 jsPlumb 实例
this.jsPlumbInstance = jsPlumb.getInstance()
// 设置连线样式
this.jsPlumbInstance.registerConnectionType('basic', {
anchor: 'Continuous',
connector: 'StateMachine'
})
},
methods: {
handleMouseDown(e) {
// 获取当前鼠标的位置,作为起始点
this.startPoint = {
x: e.clientX,
y: e.clientY
}
// 创建一个临时的连线元素
this.tempConnection = this.jsPlumbInstance.connect({
source: 'canvas',
target: 'canvas',
type: 'basic',
paintStyle: {
strokeWidth: 2,
stroke: '#000'
}
})
},
handleMouseMove(e) {
if (this.tempConnection) {
// 获取当前鼠标的位置,作为结束点
const endPoint = {
x: e.clientX,
y: e.clientY
}
// 更新连线的起始点和结束点
this.jsPlumbInstance.setSuspendDrawing(true)
this.jsPlumbInstance.deleteConnection(this.tempConnection)
this.tempConnection = this.jsPlumbInstance.connect({
source: this.startPoint,
target: endPoint,
type: 'basic',
paintStyle: {
strokeWidth: 2,
stroke: '#000'
}
})
this.jsPlumbInstance.setSuspendDrawing(false, true)
}
},
handleMouseUp(e) {
if (this.tempConnection) {
// 将起始点和结束点之间的连线保存到数据中
const endPoint = {
x: e.clientX,
y: e.clientY
}
this.connections.push({
source: this.startPoint,
target: endPoint
})
// 销毁临时的连线元素
this.jsPlumbInstance.deleteConnection(this.tempConnection)
this.tempConnection = null
}
}
}
}
</script>
```
阅读全文
相关推荐
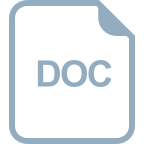
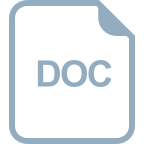
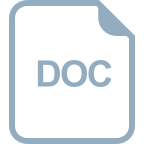

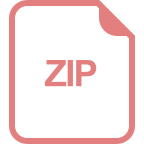
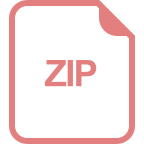
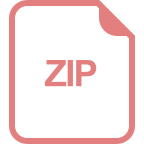
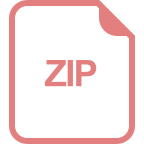



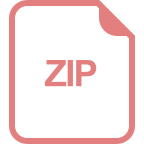
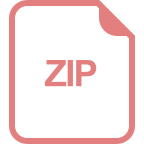
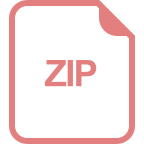
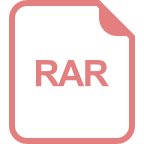
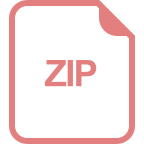
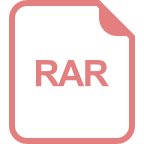
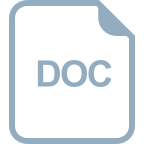
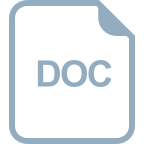