def aug(src): """图像亮度增强""" if get_lightness(src) > 130: print("图片亮度足够,不做增强") # 先计算分位点,去掉像素值中少数异常值,这个分位点可以自己配置。 # 比如1中直方图的红色在0到255上都有值,但是实际上像素值主要在0到20内。 max_percentile_pixel, min_percentile_pixel = compute(src, 1, 99) # 去掉分位值区间之外的值 src[src >= max_percentile_pixel] = max_percentile_pixel src[src <= min_percentile_pixel] = min_percentile_pixel # 将分位值区间拉伸到0到255,这里取了2550.1与2550.9是因为可能会出现像素值溢出的情况,所以最好不要设置为0到255。 out = np.zeros(src.shape, src.dtype) cv2.normalize(src, out, 255 * 0.1, 255 * 0.9, cv2.NORM_MINMAX) return out 这个代码如何修改为单个文件代码对图片进行图像增强
时间: 2024-02-11 15:09:30 浏览: 24
你可以将这段代码整合到一个单独的Python文件中,然后添加读取图片和保存图片的代码,以实现对单个图片的图像增强。修改后的代码如下:
```
import cv2
import numpy as np
def get_lightness(src):
"""计算图像亮度"""
hsv = cv2.cvtColor(src, cv2.COLOR_BGR2HSV)
h, s, v = cv2.split(hsv)
return np.mean(v)
def compute(src, low_percentile, high_percentile):
"""计算分位点"""
hist, bins = np.histogram(src.flatten(), 256, [0, 256])
cdf = hist.cumsum()
cdf_normalized = cdf / cdf.max()
low_limit = np.percentile(cdf_normalized, low_percentile)
high_limit = np.percentile(cdf_normalized, high_percentile)
min_gray = np.argwhere(cdf_normalized >= low_limit)[0]
max_gray = np.argwhere(cdf_normalized <= high_limit)[-1]
return max_gray, min_gray
def aug(src_path, dst_path):
"""图像亮度增强"""
src = cv2.imread(src_path)
if get_lightness(src) > 130:
print("图片亮度足够,不做增强")
return
# 先计算分位点,去掉像素值中少数异常值,这个分位点可以自己配置。
# 比如1中直方图的红色在0到255上都有值,但是实际上像素值主要在0到20内。
max_percentile_pixel, min_percentile_pixel = compute(src, 1, 99)
# 去掉分位值区间之外的值
src[src >= max_percentile_pixel] = max_percentile_pixel
src[src <= min_percentile_pixel] = min_percentile_pixel
# 将分位值区间拉伸到0到255,这里取了255*0.1与255*0.9是因为可能会出现像素值溢出的情况,所以最好不要设置为0到255。
out = np.zeros(src.shape, src.dtype)
cv2.normalize(src, out, 255 * 0.1, 255 * 0.9, cv2.NORM_MINMAX)
# 保存增强后的图片
cv2.imwrite(dst_path, out)
# 测试
img_path = 'test.jpg'
aug(img_path, 'test_aug.jpg')
```
在测试代码中,`img_path`是原始图片路径,'test_aug.jpg'是增强后的图片路径。注意,这里只对单张图片进行增强,如果你需要对整个文件夹中的图片进行增强,需要使用`os`模块来批量读取和保存图片。
相关推荐
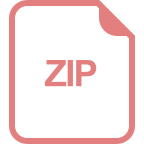
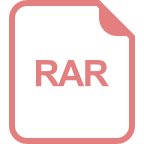
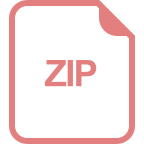














