自行实现失焦模糊代码python
时间: 2023-07-23 21:11:37 浏览: 100
在Python中,你也可以自行实现失焦模糊效果,下面是一个基本的实现示例:
```python
from PIL import Image
def blur(image, radius):
# 创建一张模糊后的图片
blurred_image = Image.new('RGBA', image.size, (0, 0, 0, 0))
pixels = blurred_image.load()
# 遍历每个像素,计算模糊值并赋值
for i in range(image.size[0]):
for j in range(image.size[1]):
r_total, g_total, b_total, a_total = 0, 0, 0, 0
count = 0
for x in range(i-radius, i+radius+1):
for y in range(j-radius, j+radius+1):
if x >= 0 and x < image.size[0] and y >= 0 and y < image.size[1]:
r, g, b, a = image.getpixel((x, y))
r_total += r
g_total += g
b_total += b
a_total += a
count += 1
if count > 0:
r_avg = int(r_total / count)
g_avg = int(g_total / count)
b_avg = int(b_total / count)
a_avg = int(a_total / count)
pixels[i, j] = (r_avg, g_avg, b_avg, a_avg)
return blurred_image
# 使用示例
img = Image.open('example.jpg')
blurred_img = blur(img, 5)
blurred_img.save('blurred_example.jpg')
```
在上面的代码中,我们定义了一个`blur`函数,它接受一个`Image`对象和一个模糊半径。该函数首先创建了一张空白的`RGBA`图片,然后遍历原始图片中的每个像素,计算模糊后的像素值并赋值到新图片中。
使用该函数时,我们首先打开了一张名为`example.jpg`的图片。然后调用`blur`函数,将原始图片和半径5作为参数传递给它,得到一张模糊后的图片。最后,我们将模糊后的图像保存为`blurred_example.jpg`。
需要注意的是,由于该函数是基于遍历每个像素来实现的,因此它可能比使用`ImageFilter.BLUR`更慢,尤其是对于大型图像。如果需要处理大型图像,建议使用更高效的算法。
阅读全文
相关推荐
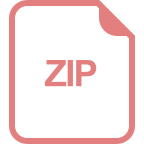
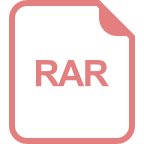
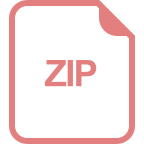

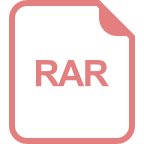
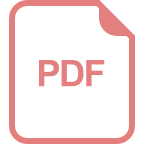
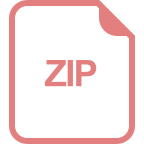
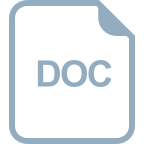
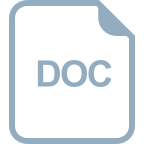
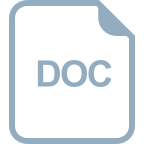
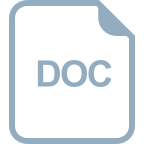
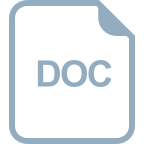
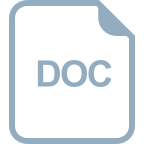
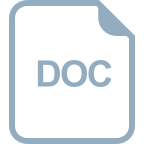




