c# 中 使用ML.NET结合Tensorflow问答机器人的代码
时间: 2024-02-17 16:01:34 浏览: 134
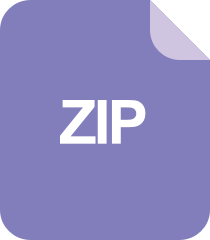
c#调用tensorflow的例子

以下是使用 ML.NET 和 TensorFlow 实现问答机器人的示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.IO;
using Microsoft.ML;
using Microsoft.ML.Data;
using TensorFlow;
namespace QABot
{
class Program
{
static void Main(string[] args)
{
// 加载数据
var dataView = LoadData("qa_data.txt");
// 定义模型架构
var pipeline = new LearningPipeline();
pipeline.Add(new TextFeaturizer("Features", "Question"));
pipeline.Add(new TensorFlowTransformer
{
ModelLocation = "qa_model.pb",
Inputs = new[] { "Features" },
Outputs = new[] { "Score" }
});
pipeline.Add(new ColumnCopier(("Score", "Prediction")));
pipeline.Add(new PredictedLabelColumnOriginalValueConverter
{
PredictedLabelColumn = "Prediction",
OriginalLabelColumn = "Answer",
});
// 训练模型
var model = pipeline.Train<QAData, QAPrediction>(dataView);
// 使用模型进行预测
var prediction = model.Predict(new QAData { Question = "What is your name?" });
Console.WriteLine($"Q: {prediction.Question}");
Console.WriteLine($"A: {prediction.Answer}");
Console.ReadKey();
}
private static IDataView LoadData(string path)
{
var lines = File.ReadAllLines(path);
var data = new List<QAData>();
foreach (var line in lines)
{
var parts = line.Split('\t');
data.Add(new QAData { Question = parts[0], Answer = parts[1] });
}
var context = new MLContext();
return context.Data.LoadFromEnumerable(data);
}
}
public class QAData
{
[Column("0")]
public string Question { get; set; }
[Column("1")]
public string Answer { get; set; }
}
public class QAPrediction
{
[ColumnName("Answer")]
public string Answer { get; set; }
[ColumnName("Prediction")]
public float[] Score { get; set; }
[ColumnName("Features")]
public float[] Features { get; set; }
}
}
```
上述代码中,我们首先加载问答数据,然后使用 ML.NET 定义模型架构,包括文本特征提取、使用 TensorFlow 进行预测等步骤。接着,我们使用数据训练模型,并使用模型进行预测。
需要注意的是,上述代码中的 `qa_data.txt` 和 `qa_model.pb` 文件需要根据实际情况进行替换。同时,需要将 TensorFlow 模型转换为 ML.NET 模型,可以使用以下代码:
```csharp
var tfModel = File.ReadAllBytes("qa_model.pb");
var mlModel = TensorFlowUtils.LoadTensorFlowModel(new TFModel { Model = tfModel });
mlModel.Save("qa_model.zip");
```
以上代码将 TensorFlow 模型转换为 ML.NET 模型,并将其保存为 `qa_model.zip` 文件。
阅读全文
相关推荐
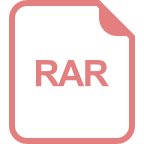
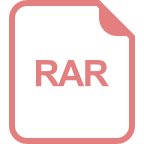
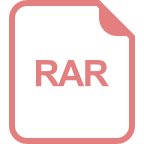
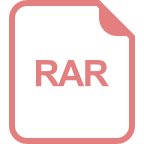
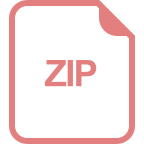
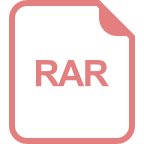
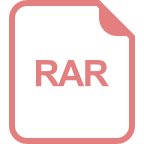
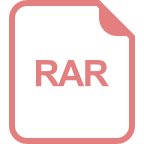
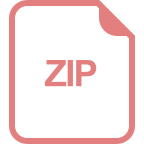
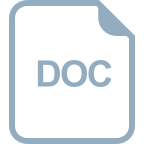
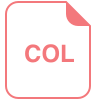
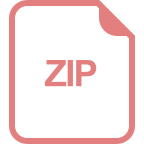
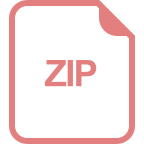
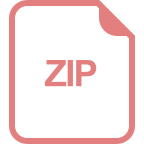